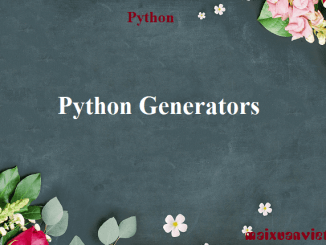
Python Generators
In this tutorial, you’ll learn how to create iterations easily using Python generators, how it is different from iterators and normal functions, and why you […]
In this tutorial, you’ll learn how to create iterations easily using Python generators, how it is different from iterators and normal functions, and why you […]
Iterators are objects that can be iterated upon. In this tutorial, you will learn how iterator works and how you can build your own iterator […]
You can change the meaning of an operator in Python depending upon the operands used. In this tutorial, you will learn how to use operator […]
In this tutorial, you’ll learn about multiple inheritance in Python and how to use it in your program. You’ll also learn about multi-level inheritance and […]
Inheritance enables us to define a class that takes all the functionality from a parent class and allows us to add more. In this tutorial, […]
In this tutorial, you will learn about the core functionality of Python objects and classes. You’ll learn what a class is, how to create it […]
In this tutorial, you’ll learn about Object-Oriented Programming (OOP) in Python and its fundamental concept with the help of examples. 1. Object Oriented Programming Python […]
In this tutorial, you will learn how to define custom exceptions depending upon your requirements with the help of examples. Python has numerous built-in exceptions that force […]
In this tutorial, you’ll learn how to handle exceptions in your Python program using try, except and finally statements with the help of examples. 1. […]
In this tutorial, you will learn about different types of errors and exceptions that are built-in to Python. They are raised whenever the Python interpreter […]
In this tutorial, you’ll learn about file and directory management in Python, i.e. creating a directory, renaming it, listing all directories, and working with them. […]
In this tutorial, you’ll learn about Python file operations. More specifically, opening a file, reading from it, writing into it, closing it, and various file […]
In this tutorial, you’ll learn everything about Python dictionaries; how they are created, accessing, adding, removing elements from them and various built-in methods. Python dictionary […]
In this tutorial, you’ll learn everything about Python sets; how they are created, adding or removing elements from them, and all operations performed on sets […]
In this tutorial you will learn to create, format, modify and delete strings in Python. Also, you will be introduced to various string operations and […]
In this article, you’ll learn everything about Python tuples. More specifically, what are tuples, how to create them, when to use them and various methods […]
In this tutorial, we’ll learn everything about Python lists, how they are created, slicing of a list, adding or removing elements from them and so […]
In this article, you’ll learn about the different numbers used in Python, how to convert from one data type to the other, and the mathematical […]
In this article, you’ll learn to divide your code base into clean, efficient modules using Python packages. Also, you’ll learn to import and use your […]
In this article, you will learn to create and import custom modules in Python. Also, you will find different techniques to import and use custom […]