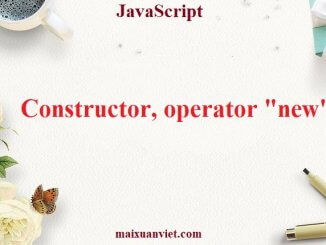
JavaScript Constructor, operator “new”
The regular {…} syntax allows to create one object. But often we need to create many similar objects, like multiple users or menu items and so on. […]
The regular {…} syntax allows to create one object. But often we need to create many similar objects, like multiple users or menu items and so on. […]
Objects are usually created to represent entities of the real world, like users, orders and so on: And, in the real world, a user can act: […]
Memory management in JavaScript is performed automatically and invisibly to us. We create primitives, objects, functions… All that takes memory. What happens when something is […]
1. Overview One of the fundamental differences of objects versus primitives is that objects are stored and copied “by reference”, whereas primitive values: strings, numbers, […]
As we know from the chapter Data types, there are eight data types in JavaScript. Seven of them are called “primitive”, because their values contain only […]
The JavaScript language steadily evolves. New proposals to the language appear regularly, they are analyzed and, if considered worthy, are appended to the list at ecma262 and […]
Automated testing will be used in further tasks, and it’s also widely used in real projects. 1. Why do we need tests? When we write […]
Learning without thought is labor lost; thought without learning is perilous. Programmer ninjas of the past used these tricks to sharpen the mind of code […]
As we know from the chapter Code structure, comments can be single-line: starting with // and multiline: /* … */. We normally use them to describe how and why […]
Our code must be as clean and easy to read as possible. That is actually the art of programming – to take a complex task […]
Before writing more complex code, let’s talk about debugging. Debugging is the process of finding and fixing errors within a script. All modern browsers and most […]
This chapter briefly recaps the features of JavaScript that we’ve learned by now, paying special attention to subtle moments. 1. Code structure Statements are delimited […]
1. Overview There’s another very simple and concise syntax for creating functions, that’s often better than Function Expressions. It’s called “arrow functions”, because it looks […]
1. Overview In JavaScript, a function is not a “magical language structure”, but a special kind of value. The syntax that we used before is […]
Quite often we need to perform a similar action in many places of the script. For example, we need to show a nice-looking message when […]
A switch statement can replace multiple if checks. It gives a more descriptive way to compare a value with multiple variants. 1. The syntax The switch has one or more case blocks and […]
We often need to repeat actions. For example, outputting goods from a list one after another or just running the same code for each number […]
1. Overview A recent additionThis is a recent addition to the language. Old browsers may need polyfills. The nullish coalescing operator is written as two […]
There are four logical operators in JavaScript: || (OR), && (AND), ! (NOT), ?? (Nullish Coalescing). Here we cover the first three, the ?? operator is in the next article. Although they are called “logical”, […]
Sometimes, we need to perform different actions based on different conditions. To do that, we can use the if statement and the conditional operator ?, that’s also called […]