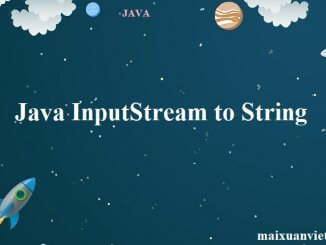
Java InputStream to String
1. Overview In this tutorial, we’ll look at how to convert an InputStream to a String. We’ll start by using plain Java, including Java8/9 solutions, then look into […]
1. Overview In this tutorial, we’ll look at how to convert an InputStream to a String. We’ll start by using plain Java, including Java8/9 solutions, then look into […]
1. Introduction Converting char to String instances is a very common operation. In this article, we will show multiple ways of tackling this situation. 2. String.valueOf() The String class has a […]
1. Introduction Converting a String to an int or Integer is a very common operation in Java. In this article, we will show multiple ways of dealing with this issue. There […]
1. Overview When dealing with Strings in Java, sometimes we need to encode them into a specific charset. This tutorial is a practical guide showing different […]
1. Overview In this short tutorial, we’re going to look at converting an array of strings or integers to a string and back again. We […]
1. Introduction We frequently need to convert between String and byte array in Java. In this tutorial, we’ll examine these operations in detail. First, we’ll look at various ways […]
1. Overview In this quick tutorial, we’ll see how to convert a ZonedDateTime to a String. We’ll also look at how to parse a ZonedDateTime from a String. 2. Creating a ZonedDateTime […]
1. Introduction Oftentimes while operating upon Strings, we need to figure out whether a String is a valid number or not. In this tutorial, we’ll explore multiple ways to […]
1. Introduction In this quick article, we’ll have a look at how to convert a List of elements to a String. This might be useful in certain scenarios […]
1. Overview Java 8 introduced the Stream API, with functional-like operations for processing sequences. If you want to read more about it, have a look at this article. […]
1. Introduction When dealing with exceptions in Java, we’re frequently logging or simply displaying stack traces. However, sometimes, we don’t want just to print the […]
1. Overview In this quick article, we’ll explore some simple conversions of String objects to different data types supported in Java. 2. Converting String to int or Integer If we need to […]
1. Introduction Java String is one of the most important classes and we’ve already covered a lot of its aspects in our String-related series of tutorials. In this […]
1. Overview String formatting and generating text output often comes up during programming. In many cases, there is a need to add a new line […]
1. Introduction Simply put, CharSequence and String are two different fundamental concepts in Java. In this quick article, we’re going to have a look at the differences between these […]
1. Introduction Splitting Strings is a very frequent operation; this quick tutorial is focused on some of the API we can use to do this simply in […]
1. Introduction In this article, we’re going to see how we can check whether a given String is a palindrome using Java. A palindrome is a word, […]
1. Overview There are many ways to count the number of occurrences of a char in a String in Java. In this quick tutorial, we’ll focus on […]
1. Overview In this quick article, we are going to check and discuss different techniques for removing the last character of a String. 2. Using String.substring() The […]
1. Introduction In this tutorial, we’re going to learn how to generate a random string in Java, first using the standard Java libraries, then using […]