Table of Contents
The isdisjoint() method returns True if two sets are disjoint sets. If not, it returns False.
Two sets are said to be disjoint sets if they have no common elements. For example:
A = {1, 5, 9, 0} B = {2, 4, -5}
Here, sets A and B are disjoint sets.
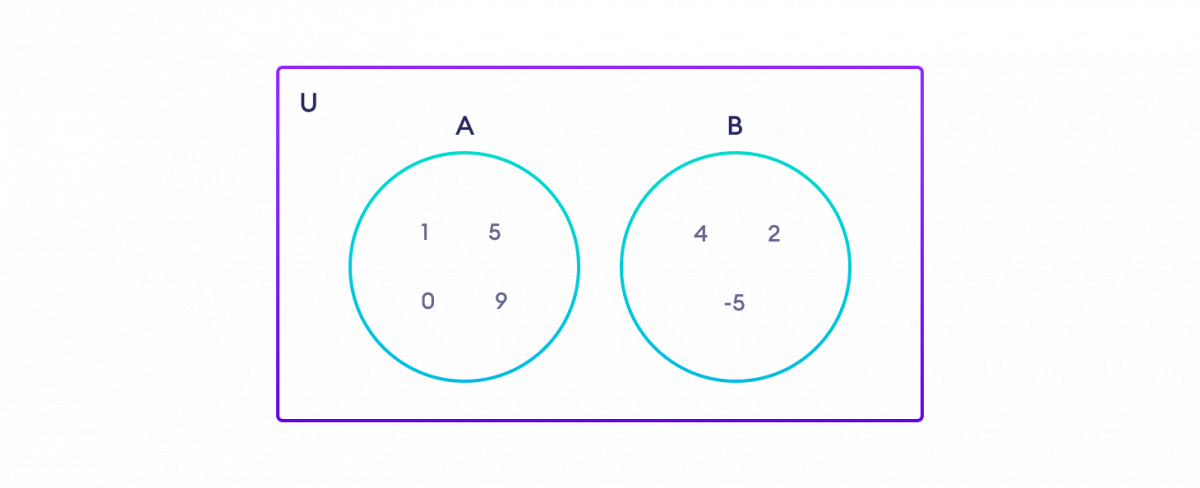
The syntax of isdisjoint()
is:
set_a.isdisjoint(set_b)
1. isdisjoint() Parameters
isdisjoint()
method takes a single argument (a set).
You can also pass an iterable (list, tuple, dictionary, and string) to disjoint()
. isdisjoint()
method will automatically convert iterables to set and checks whether the sets are disjoint or not.
2. Return Value from isdisjoint()
isdisjoint()
method returns
True
if two sets are disjoint sets (if set_a and set_b are disjoint sets in above syntax)False
if two sets are not disjoint sets
3. Example 1: How isdisjoint() works?
A = {1, 2, 3, 4} B = {5, 6, 7} C = {4, 5, 6} print('Are A and B disjoint?', A.isdisjoint(B)) print('Are A and C disjoint?', A.isdisjoint(C))
Output
Are A and B disjoint? True Are A and C disjoint? False
4. Example 2: isdisjoint() with Other Iterables as arguments
A = {'a', 'b', 'c', 'd'} B = ['b', 'e', 'f'] C = '5de4' D ={1 : 'a', 2 : 'b'} E ={'a' : 1, 'b' : 2} print('Are A and B disjoint?', A.isdisjoint(B)) print('Are A and C disjoint?', A.isdisjoint(C)) print('Are A and D disjoint?', A.isdisjoint(D)) print('Are A and E disjoint?', A.isdisjoint(E))
Output
Are A and B disjoint? False Are A and C disjoint? False Are A and D disjoint? True Are A and E disjoint? False
Related posts:
Python Operator Overloading
Python tuple()
Python List count()
Python Program to Create Pyramid Patterns
Python sorted()
Python Dictionary copy()
Python Program to Find Armstrong Number in an Interval
Python Artificial Intelligence Project for Beginners - Joshua Eckroth
Python Program to Find All File with .txt Extension Present Inside a Directory
Python Program to Count the Number of Each Vowel
Python zip()
Python ascii()
Python len()
Python Program to Convert Celsius To Fahrenheit
Python Program to Merge Mails
Python List clear()
Python Program to Convert Decimal to Binary Using Recursion
Python Program to Compute all the Permutation of the String
Python callable()
Python Operators
Python strftime()
Python Tuple count()
Python Program to Add Two Numbers
Learning scikit-learn Machine Learning in Python - Raul Garreta & Guillermo Moncecchi
Python reversed()
Introduction to Machine Learning with Python - Andreas C.Muller & Sarah Guido
Python 3 for Absolute Beginners - Tim Hall & J.P Stacey
Node.js vs Python for Backend Development
Python List
Python Program to Split a List Into Evenly Sized Chunks
Python *args and **kwargs
Python for Programmers with introductory AI case studies - Paul Deitel & Harvey Deitel