Table of Contents
In this tutorial, we will learn about the Python String find() method with the help of examples.
The find()
method returns the index of first occurrence of the substring (if found). If not found, it returns -1.
Example
message = 'Python is a fun programming language' # check the index of 'fun' print(message.find('fun')) # Output: 12
1. Syntax of String find()
The syntax of the find()
method is:
str.find(sub[, start[, end]] )
2. find() Parameters
The find()
method takes maximum of three parameters:
- sub – It is the substring to be searched in the str string.
- start and end (optional) – The range
str[start:end]
within which substring is searched.
3. find() Return Value
The find()
method returns an integer value:
- If the substring exists inside the string, it returns the index of the first occurence of the substring.
- If a substring doesn’t exist inside the string, it returns -1.
4. Working of find() method
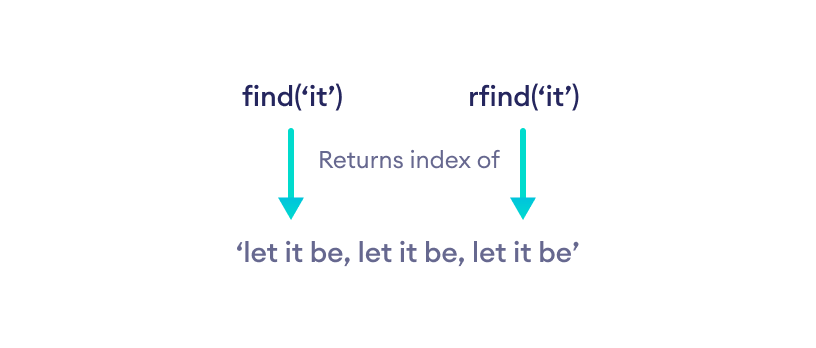
5. Example 1: find() With No start and end Argument
quote = 'Let it be, let it be, let it be' # first occurance of 'let it'(case sensitive) result = quote.find('let it') print("Substring 'let it':", result) # find returns -1 if substring not found result = quote.find('small') print("Substring 'small ':", result) # How to use find() if (quote.find('be,') != -1): print("Contains substring 'be,'") else: print("Doesn't contain substring")
Output
Substring 'let it': 11 Substring 'small ': -1 Contains substring 'be,'
6. Example 2: find() With start and end Arguments
quote = 'Do small things with great love' # Substring is searched in 'hings with great love' print(quote.find('small things', 10)) # Substring is searched in ' small things with great love' print(quote.find('small things', 2)) # Substring is searched in 'hings with great lov' print(quote.find('o small ', 10, -1)) # Substring is searched in 'll things with' print(quote.find('things ', 6, 20))
Output
-1 3 -1 9
Related posts:
JavaScript Eval: run a code string
Machine Learning with Python for everyone - Mark E.Fenner
Python Statement, Indentation and Comments
Python List remove()
Python zip()
Python Set union()
Python getattr()
Python String istitle()
Python String count()
Python String lower()
Python Anonymous / Lambda Function
Python Program to Check If Two Strings are Anagram
Python format()
Python Set difference_update()
Python Recursion
Python String isnumeric()
Machine Learning Mastery with Python - Understand your data, create accurate models and work project...
Python Deeper Insights into Machine Learning - Sebastian Raschka & David Julian & John Hearty
Converting String to Stream of chars
Deep Learning with Applications Using Python - Navin Kumar Manaswi
Python Dictionary items()
Python Program to Sort a Dictionary by Value
Python ascii()
Python String format()
Python Program to Print Output Without a Newline
Python reversed()
Python String rstrip()
Python List Comprehension
Python slice()
Python Program to Find the Size (Resolution) of a Image
String Initialization in Java
Python Program to Check Whether a String is Palindrome or Not