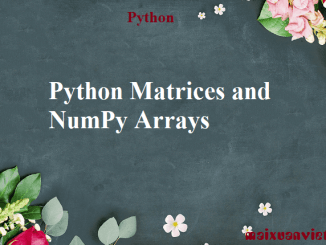
Python Matrices and NumPy Arrays
In this article, we will learn about Python matrices using nested lists, and NumPy package. A matrix is a two-dimensional data structure where numbers are […]
In this article, we will learn about Python matrices using nested lists, and NumPy package. A matrix is a two-dimensional data structure where numbers are […]
In this example, you will learn to transpose a matrix (which is created by using a nested list). To understand this example, you should have […]
In this program, you’ll learn to add two matrices using Nested loop and Next list comprehension, and display it. To understand this example, you should […]
In this program, you will learn to convert decimal number to binary using recursive function. o understand this example, you should have the knowledge of […]
In this program, you’ll learn to find the factorial of a number using recursive function. To understand this example, you should have the knowledge of […]
In this program, you’ll learn to find the sum of natural numbers using recursive function. To understand this example, you should have the knowledge of […]
To understand this example, you should have the knowledge of the following Python programming topics: Python for Loop Python Functions Python Recursion A Fibonacci sequence is the […]
Python has a built-in function, calendar to work with date related tasks. You will learn to display the calendar of a given date in this […]
In this program, you’ll learn to shuffle a deck of cards using random module. To understand this example, you should have the knowledge of the […]
In this example you will learn to create a simple calculator that can add, subtract, multiply or divide depending upon the input from the user. […]
In this program, you’ll learn to find the factors of a number using the for loop. To understand this example, you should have the knowledge […]
In this program, you’ll learn to find the LCM of two numbers and display it. To understand this example, you should have the knowledge of […]
In this example, you will learn to find the GCD of two numbers using two different methods: function and loops and, Euclidean algorithm To understand […]
In this program, you’ll learn to find the ASCII value of a character and display it. To understand this example, you should have the knowledge […]
In this program, you’ll learn to convert decimal to binary, octal and hexadecimal, and display it. To understand this example, you should have the knowledge […]
In this program, you’ll learn to find the numbers divisible by another number and display it. To understand this example, you should have the knowledge […]
In this program, you’ll learn to display powers of the integer 2 using Python anonymous function. To understand this example, you should have the knowledge […]
In this program, you’ll learn to find the sum of n natural numbers using while loop and display it. To understand this example, you should […]
In this example, we will learn to find all the Armstrong numbers present in between two intervals in Python. To understand this example, you should […]
In this example, you will learn to check whether an n-digit integer is an Armstrong number or not. To understand this example, you should have […]