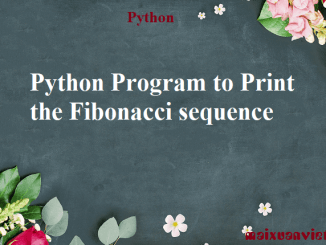
Python Program to Print the Fibonacci sequence
In this program, you’ll learn to print the Fibonacci sequence using while loop. To understand this example, you should have the knowledge of the following Python […]
In this program, you’ll learn to print the Fibonacci sequence using while loop. To understand this example, you should have the knowledge of the following Python […]
This program displays the multiplication table of variable num (from 1 to 10). To understand this example, you should have the knowledge of the following Python […]
In this article, you’ll learn to find the factorial of a number and display it. To understand this example, you should have the knowledge of […]
In this program, you’ll learn to print all prime numbers within an interval using for loops and display it. To understand this example, you should […]
Example to check whether an integer is a prime number or not using for loop and if…else statement. If the number is not prime, it’s […]
In this program, you’ll learn to find the largest among three numbers using if else and display it. To understand this example, you should have […]
In this program, you will learn to check whether a year is leap year or not. We will use nested if…else to solve this problem. […]
In this example, you will learn to check whether a number entered by the user is even or odd. To understand this example, you should […]
In this example, you will learn to check whether a number entered by the user is positive, negative or zero. This problem is solved using […]
In this program, you’ll learn to convert Celsuis to Fahrenheit and display it. To understand this example, you should have the knowledge of the following Python […]
In this example, we’ll learn to convert kilometers to miles and display it. To understand this example, you should have the knowledge of the following Python […]
In this example, you will learn to generate a random number in Python. To understand this example, you should have the knowledge of the following Python […]
In this example, you will learn to swap two variables by using a temporary variable and, without using temporary variable. To understand this example, you […]
This program computes roots of a quadratic equation when coefficients a, b and c are known. To understand this example, you should have the knowledge […]
In this program, you’ll learn to calculate the area of a triangle and display it. To understand this example, you should have the knowledge of […]
In this program, you’ll learn to find the square root of a number using exponent operator and cmath module. To understand this example, you should […]
In this program, you will learn to add two numbers and display it using print() function. To understand this example, you should have the knowledge […]
A simple program that displays “Hello, World!”. It’s often used to illustrate the syntax of the language. To understand this example, you should have the […]
The sleep() function suspends (waits) execution of the current thread for a given number of seconds. Python has a module named time which provides several useful functions […]
1. Overview In this article, we will explore time module in detail. We will learn to use different time-related functions defined in the time module […]