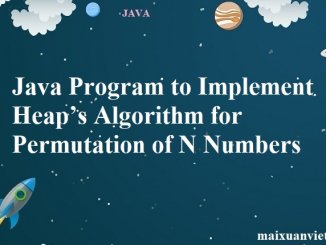
Java Program to Implement Heap’s Algorithm for Permutation of N Numbers
This is a java program to find permutation of N numbers using Heap’s Algorithm. Heap’s algorithm is an algorithm used for generating all possible permutations […]
This is a java program to find permutation of N numbers using Heap’s Algorithm. Heap’s algorithm is an algorithm used for generating all possible permutations […]
This is a java program to perform quick sort with complexity constraint of time less than n^2. Here is the source code of the Java […]
This is a Java Program to Implement Interpolation Search Algorithm. Interpolation search (sometimes referred to as extrapolation search) is an algorithm for searching for a […]
This is a Java Program to implement Threaded Binary Tree. A threaded binary tree makes it possible to traverse the values in the binary tree […]
This is a java program to search sequence using binary search. This is a simple extension of binary search algorithm to find an element. Here […]
This is a Java Program to Implement Ternary Search Algorithm. A ternary search algorithm is a technique in computer science for finding the minimum or […]
This is a Java Program to Implement Interpolation Search Algorithm. Interpolation search (sometimes referred to as extrapolation search) is an algorithm for searching for a […]
This is a Java Program to implement Counting Sort Algorithm. This program is to sort a list of numbers.Here is the source code of the […]
This is a java program to implement merge sort algorithm using linked list. Here is the source code of the Java Program to Implement Merge […]
This is a java program to sort an elements in order n time. Bucket sort can be used to achieve this goal. Bucket sort is […]
Problem Description Given an array of integers, sort the array using heapsort algorithm, as built into the library. Problem Solution The idea is to create a […]
This is a java program to implement Shell Sort Algorithm. Here is the source code of the Java Program to Implement Shell Sort. The Java […]
Problem Description Given an array of integers, sort the array using the quicksort algorithm. Problem Solution The idea is to divide the array into two parts, […]
This is a java program to perform sorting using Randomized Quick Sort. Randomized Quick Sort randomly selects a pivot element, after selecting pivot standard procedure […]
This is a Java Program to implement Selection Sort on an integer array. Selection sort is a sorting algorithm, specifically an in-place comparison sort. It […]
This is a java program to sort the numbers using the Bubble Sort Technique. The algorithm goes with the name, generally used to sort numbers […]
This is a java program to sort the numbers using the Bucket Sort Technique. The algorithm allocates the number of memory locations equal to maximum […]
This is a Java Program to implement Radix Sort Algorithm. This program is to sort a list of numbers.Here is the source code of the […]
This is a Java Program to Implement Gaussian Elimination Algorithm. Gaussian elimination (also known as row reduction) is an algorithm for solving systems of linear […]
This is a Java Program to Implement Regular Falsi Algorithm. Regular Falsi method is used for finding roots of functions. Here is the source code […]