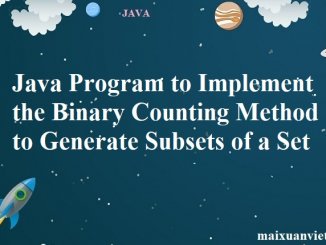
Java Program to Implement the Binary Counting Method to Generate Subsets of a Set
This is a java program to generate and print all possible subsets using the method of Binary Counting method. The generations of subsets are done […]
This is a java program to generate and print all possible subsets using the method of Binary Counting method. The generations of subsets are done […]
This is a java program to generate and print all the subsets of a given set as per lexicographical order, here we follow the numerical […]
This is a java program to generate and print all the subsets using the Gray Code Order. The reflected binary code, also known as Gray […]
This is a java program to generate sequence of N characters randomly. Here is the source code of the Java Program to Generate a Sequence […]
This is a java program to generate and print all the permutation of the Numbers. User first enters the element in the set and then […]
This is a java program to find permutation of N numbers using Heap’s Algorithm. Heap’s algorithm is an algorithm used for generating all possible permutations […]
This is the Java Program to Print all Possible Permutations of a String.Problem Description Given a string, print all the different words that can be […]
This is a java program to implement Alexander Bogomolny’s permutation algorithm. This version of program computes all possible permutations of numbers from 1 to N […]
This is a java program to find the mode of a set. The mode of a set is defined as the highest occurring element in […]
This is a java program to find kth smallest element form the given sequence of numbers. This could be solved by using Quick sort algorithm, […]
This is a java program to find the ith largest element from a list using order-statistic algorithm. This version of the code uses quick sort […]
This is a java program to find the median from two different array. To do so we merge the two lists and then sort them, […]
This is a java program to find K such elements given by users, such that those numbers are closer to the median of the given […]
This is a java program to perform quick sort with complexity constraint of time less than n^2. Here is the source code of the Java […]
This is a java program to find the second smallest element with given complexity. Complexity here is minimum space constraints. Inplace sorting and returning second […]
This is a Java Program to find median of two sorted arrays using binary search approach. In probability theory and statistics, a median is described […]
This is a Java Program to find number of occurences of a given number using binary search approach. The time complexity of the following program […]
Problem Description Given an array of integers, find the contiguous subarray, whose sum of the elements, is maximum.Example: Array = [2 1 3 5 -2 […]
This is a Java Program to find maximum subarray sum of an array. A subarray is a continuous portion of an array. The time complexity […]
This is a Java Program to find peak element of an array. A peak element of an array is that element which is greater than […]