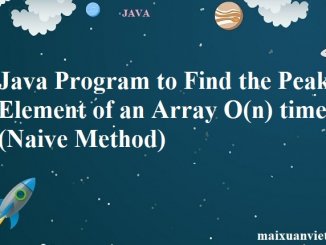
Java Program to Find the Peak Element of an Array O(n) time (Naive Method)
This is a Java Program to find peak element of an array. A peak element of an array is that element which is not smaller […]
This is a Java Program to find peak element of an array. A peak element of an array is that element which is not smaller […]
This is a Java Program to find minimum element of a rotated sorted array. The following program uses a binary search approach to find the […]
This is a java program to find the maximum element using binary search technique. Binary search requires sequence to be sorted. We return the last […]
This is a java program to find the minimum element of the sequence using the technique of linear search. First we assign min equal to […]
This is a java program to perform uniform binary search technique. It uses a lookup table to update a single array index, rather than taking […]
This is a java program to search a number using Fibonacci Sequence. The Fibonacci search technique is a method of searching a sorted array using […]
This is a java program to find kth largest element form the given sequence of numbers. We find the kth largest by sorting the sequence […]
This is a Java Program to Implement Interpolation Search Algorithm. Interpolation search (sometimes referred to as extrapolation search) is an algorithm for searching for a […]
This is a java program to perform searching based on locality of refernce. The Locality of Reference principle says that if an element of a […]
This is a Java Program to implement Threaded Binary Tree. A threaded binary tree makes it possible to traverse the values in the binary tree […]
This is a java program to search an element using Binary Search Tree. A regular tree traversal algorithm is implemented to search an element. We […]
This is a java program to search sequence using binary search. This is a simple extension of binary search algorithm to find an element. Here […]
This is a java program to search an element using Self Organizing lists. A self-organizing list is a list that reorders its elements based on […]
This is a java program to compare Binary Search and Linear Search algorithms. Following class provides the time required to search an element for both […]
This is a Java Program to Implement Ternary Search Algorithm. A ternary search algorithm is a technique in computer science for finding the minimum or […]
This is a Java Program to Implement Interpolation Search Algorithm. Interpolation search (sometimes referred to as extrapolation search) is an algorithm for searching for a […]
This is a java program to implement Stooge sort algorithm. Here is the source code of the Java Program to Perform Stooge Sort. The Java […]
This is a java program to implement Shaker Sort algorithm. Here is the source code of the Java Program to Perform the Shaker Sort. The […]
This is a java program to sort the numbers using the Counting Sort Technique. In computer science, counting sort is an algorithm for sorting a […]
This is a Java Program to implement Counting Sort Algorithm. This program is to sort a list of numbers.Here is the source code of the […]