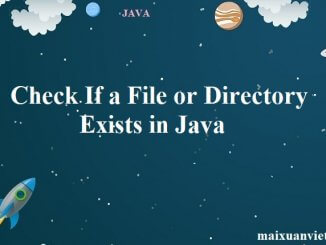
Check If a File or Directory Exists in Java
1. Overview In this quick tutorial, we’re going to get familiar with different ways to check the existence of a file or directory. First, we’ll […]
1. Overview In this quick tutorial, we’re going to get familiar with different ways to check the existence of a file or directory. First, we’ll […]
1. Overview This tutorial will show how to read all the lines from a large file in Java in an efficient manner. This article is part of the […]
1. Overview In this tutorial, we’ll take a look at various strategies for getting MIME types of a file. We’ll look at ways to extend […]
1. With Java – JDK 6 Let’s start with the standard Java 6 solution: As you can see – the file must exist before the delete […]
1. Overview In this quick tutorial, we’re going to look at renaming / moving a File in Java. We’ll first look into using the Files and Path classes from NIO, […]
1. Overview In this tutorial, we’ll explore different ways to write to a file using Java. We’ll make use of BufferedWriter, PrintWriter, FileOutputStream, DataOutputStream, RandomAccessFile, FileChannel, and the Java 7 Files utility class. We’ll also look […]
1. Overview In this tutorial, we’ll explore different ways to read from a File in Java. First, we’ll learn how to load a file from the […]
1. Overview In this quick tutorial, we’re going to learn how to create a new File in Java – first using the Files and Path classes from NIO, then […]
1. Introduction Java 8 introduced the concept of Streams to the collection hierarchy. These allow for some very powerful processing of data in a very readable way, […]
1. Overview Spring Data now supports core Java 8 features – such as Optional, Stream API and CompletableFuture. In this quick article, we’ll go through some examples of how […]
1. Introduction The Java Stream API introduces us to a powerful alternative for processing data. In this short tutorial, we’ll focus on peek(), an often misunderstood method. 2. […]
1. Introduction In this quick tutorial, we’ll examine various ways of calculating the sum of integers using the Stream API. For the sake of simplicity, we’ll use integers […]
1. Overview In this quick tutorial, we’ll discuss different ways to chain Predicates in Java 8. 2. Basic Example First, let’s see how to use a simple Predicate to filter a List of […]
1. Overview In this tutorial, we’re going to demonstrate how to implement if/else logic with Java 8 Streams. As part of the tutorial, we’ll create a simple […]
1. Overview The Spliterator interface, introduced in Java 8, can be used for traversing and partitioning sequences. It’s a base utility for Streams, especially parallel ones. In this article, […]
1. Overview In this quick write-up, we are going to focus on the new interesting Stream API improvements coming in Java 9. 2. Stream Takewhile/Dropwhile […]
1. Overview Searching for different elements in a list is one of the common tasks that we as programmers usually face. From Java 8 on […]
1. Introduction The Stream API was one of the key features added in Java 8. Briefly, the API allows us to process collections and other […]
1. Overview In this article, we will be looking at a java.util.Stream API and we’ll see how we can use that construct to operate on an infinite […]
1. Overview In this brief article, we’re going to discuss a common Exception that we may encounter when working with the Stream class in Java 8: We’ll discover the […]