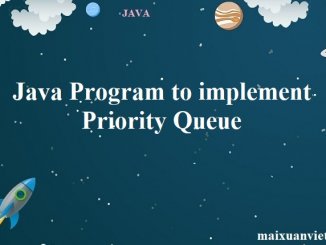
Java Program to implement Priority Queue
This is a Java Program to implement Priority Queue. A priority queue is an abstract data type which is like a regular queue or stack […]
This is a Java Program to implement Priority Queue. A priority queue is an abstract data type which is like a regular queue or stack […]
This is a Java Program to implement Bit Matrix. Here bit matrix is implemented as an array of bit sets. Here is the source code […]
This is a Java Program to implement Bit Set. Bit Set is an array data structure used to implement a simple set data structure. Here […]
This is a Java Program to implement Circular Buffer. A circular buffer, cyclic buffer or ring buffer is a data structure that uses a single, […]
This is a Java Program to implement Bi Directional Map. A bi-directional map is an associative data structure in which the (key, value) pairs form […]
This is a Java Program to implement Count Min Sketch. The Count–min sketch (or CM sketch) is a probabilistic sub-linear space streaming algorithm which can […]
This is a Java Program to implement Bloom Filter. A Bloom filter is a space-efficient probabilistic data structure that is used to test whether an […]
This Java program is to Implement Segment tree. In computer science, a segment tree is a tree data structure for storing intervals, or segments. It […]
This is a Java Program to implement Array Deque. Deque is an abstract data type that generalizes a queue, for which elements can be added […]
This is a Java Program to implement Sparse Vector. Sparse vectors are useful implementation of vectors that mostly contains zeroes. Here sparse vectors consists of […]
This is a Java Program to Implement Sorted Vector. Here Sorted vector is implemented using a vector and each inserted element is placed at correct […]
This is a Java Program to Implement Sorted List. Here Sorted list is implemented using an array list and each inserted element is placed at […]
This is a Java Program to implement Associate Array. An associative array, map, symbol table, or dictionary is an abstract data type composed of a […]
This is a Java Program to implement Dynamic Array. A dynamic array, growable array, resizable array, dynamic table, mutable array, or array list is a […]
This is a Java Program to implement Self Organizing List. A self-organizing list is a list that reorders its elements based on some self-organizing heuristic […]
This is a Java Program to implement Triply Linked List. Triply linked list is a list in which each node has 3 pointers pointing to […]
This is a Java Program to subtract two large numbers using Linked List. A linked list is a data structure consisting of a group of […]
This is a Java Program to add two large numbers using Linked List. A linked list is a data structure consisting of a group of […]
This is a Java Program to implement a Sorted Circular Doubly Linked List. A linked list is a data structure consisting of a group of […]
This is a Java Program to implement a Sorted Circular Singly Linked List. A linked list is a data structure consisting of a group of […]