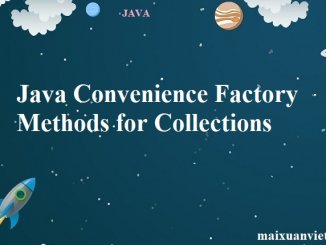
Java Convenience Factory Methods for Collections
1. Overview Java 9 brings the long-awaited syntactic sugar for creating small unmodifiable Collection instances using a concise code one-liner. As per JEP 269, new convenience factory methods […]
1. Overview Java 9 brings the long-awaited syntactic sugar for creating small unmodifiable Collection instances using a concise code one-liner. As per JEP 269, new convenience factory methods […]
1. Overview In this tutorial, we will illustrate how to concatenate multiple collections into one logical collection. We’ll be exploring five different approaches – two […]
1. Overview In this quick tutorial, we’ll learn how to join and to split Arrays and Collections in Java, making good use of the new stream support. 2. Join […]
1. Introduction In this tutorial, we’ll illustrate how to zip two collections into one logical collection. The “zip” operation is slightly different from the standard “concat” […]
1. Overview In this quick article, we’ll explore how to flatten a nested collection in Java. 2. Example of a Nested Collection Suppose we have […]
1. Overview In this quick article, we’ll see how we can shuffle a collection in Java. Java has a built-in method for shuffling List objects — we’ll utilize […]
1. Overview This article will illustrate how to apply sorting to Array, List, Set and Map in Java 7 and Java 8. 2. Sorting With Array Let’s start by sorting integer arrays […]
1. Overview In this tutorial, we’ll focus on conversion from a Map to a String and the other way around. First, we’ll see how to achieve these using core […]
1. Overview Converting List to Map is a common task. In this tutorial, we’ll cover several ways to do this. We’ll assume that each element of the List has an identifier that […]
1. Overview This short article will show how to convert the values of a Map to an Array, a List or a Set using plain Java as well as a quick Guava based example. 2. Map […]
1. Overview In this quick tutorial, we’ll take a look at the conversion between a List and a Set, starting with Plain Java, using Guava and the Apache Commons Collections library, and […]
1. Overview In this short article we’re going to look at converting between an array and a Set – first using plain java, then Guava and the Commons Collections library […]
1. Overview In this quick tutorial, we’re going to learn how to convert between an Array and a List using core Java libraries, Guava and Apache Commons […]
1. Overview In this tutorial, we’ll walk through some of the main implementations of concurrent queues in Java. For a general introduction to queues, refer […]
1. Introduction In this tutorial, we’ll be discussing Java’s Queue interface. First, we’ll take a peek at what a Queue does, and some of its core methods. Next, we’ll dive into a […]
1. Overview In this tutorial, we’ll show how to use the Java’s ArrayDeque class – which is an implementation of Deque interface. An ArrayDeque (also known as an “Array Double Ended […]
1. Overview In this article, we’ll be looking at the TransferQueue construct from the standard java.util.concurrent package. Simply put, this queue allows us to create programs according to the […]
1. Overview In this article, we’ll be looking at the SynchronousQueue from the java.util.concurrent package. Simply put, this implementation allows us to exchange information between threads in a thread-safe […]
1. Introduction In this article, we’ll focus on the PriorityBlockingQueue class and go over some practical examples. Starting with the assumption that we already know what a Queue is, […]
1. Overview We often use maps to store a collection of key-value pairs. Then, at some point, we often need to iterate over them. In this […]