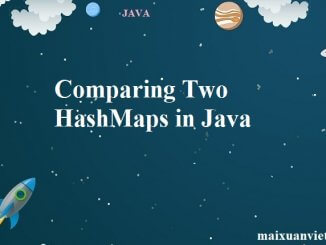
Comparing Two HashMaps in Java
1. Overview In this tutorial, we’re going to explore different ways to compare two HashMaps in Java. We’ll discuss multiple ways to check if two HashMaps are similar. We’ll also […]
1. Overview In this tutorial, we’re going to explore different ways to compare two HashMaps in Java. We’ll discuss multiple ways to check if two HashMaps are similar. We’ll also […]
1. Introduction In this quick tutorial, we’ll learn how to sort a HashMap in Java. More specifically, we’ll look at sorting HashMap entries by their key or value using: TreeMap […]
1. Introduction In this quick tutorial, we’ll demonstrate how to merge two maps using the Java 8 capabilities. To be more specific, we’ll examine different merging scenarios […]
1. Overview In this tutorial, we’ll learn about various ways of initializing a HashMap in Java. We’ll use Java 8 as well as Java 9. 2. The […]
1. Overview In this tutorial, we’re going to explore the available options for handling a Map with duplicate keys or, in other words, a Map which allows storing multiple […]
1. Overview Map is one of the most common data structures in Java, and String is one of the most common types for a map’s key. By default, a map […]
1. Overview In this article, we’ll see how to use HashMap in Java, and we’ll look at how it works internally. A class very similar to HashMap is Hashtable. Please […]
1. Overview It is sometimes preferable to disallow modifications to the java.util.Map such as sharing read-only data across threads. For this purpose, we can use either an […]
1. Overview In this article, we are going to explore the internal implementation of LinkedHashMap class. LinkedHashMap is a common implementation of Map interface. This particular implementation is a subclass of HashMap and […]
1. Overview In this article, we will be looking at a WeakHashMap from the java.util package. In order to understand the data structure, we’ll use it here to roll […]
1. Introduction In this article, we’re going to compare two Map implementations: TreeMap and HashMap. Both implementations form an integral part of the Java Collections Framework and store data as key-value pairs. 2. Differences […]
1. Overview In this article, we are going to explore TreeMap implementation of Map interface from Java Collections Framework(JCF). TreeMap is a map implementation that keeps its entries sorted according […]
1. Overview In this article, we’ll see how to use HashMap in Java, and we’ll look at how it works internally. A class very similar to HashMap is Hashtable. Please […]
1. Overview In this article, we’ll dive into HashSet. It’s one of the most popular Set implementations as well as an integral part of the Java Collections Framework. 2. […]
1. Overview In this article, we’ll have a look at an integral part of the Java Collections Framework and one of the most popular Set implementations – the TreeSet. […]
1. Introduction In this tutorial, we’ll implement two linked list reversal algorithms in Java. 2. Linked List Data Structure A linked list is a linear data structure in […]
1. Overview Finding differences between collections of objects of the same data type is a common programming task. As an example, imagine we have a […]
1. Overview In this tutorial, we’ll learn how to retrieve the intersection of two Lists. Like many other things, this has become much easier thanks to the introduction […]
1. Introduction Iterating over the elements of a list is one of the most common tasks in a program. In this tutorial, we’re going to […]
1. Overview of ArrayList In this quick tutorial, we’ll show to how to add multiple items to an already initialized ArrayList. For an introduction to the use […]