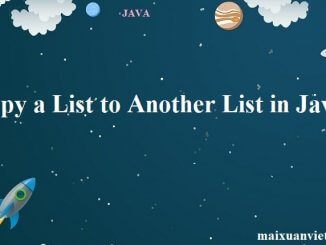
Copy a List to Another List in Java
1. Overview In this quick tutorial, we’ll show different ways to copy a List to another List and a common error produced in the process. For an introduction to […]
1. Overview In this quick tutorial, we’ll show different ways to copy a List to another List and a common error produced in the process. For an introduction to […]
1. Overview In this quick tutorial, we’ll discuss a common Exception that can occur when working with some the API of most List implementations – the UnsupportedOperationException. A java.util.List has more functionality than […]
1. Overview Finding an element in a list is a very common task we come across as developers. In this quick tutorial, we’ll cover different […]
1. Introduction In this short article we’ll focus on the common problem of testing if two List instances contain the same elements in exactly the same order. […]
This quick tutorial is going to show how to remove all null elements from a List, using plain Java, Guava, the Apache Commons Collections and the newer Java 8 lambda […]
In this quick tutorial we’re going to show how to clean up the duplicate elements from a List – first using plain Java, then Guava, and finally […]
1. Remove Nulls From a List Using Plain Java The Java Collections Framework offers a simple solution for removing all null elements in the List – a basic while loop: Alternatively, we […]
1. Overview In this tutorial I will illustrate how to split a List into several sublists of a given size. For a relatively simple operation, there is […]
1. Introduction Picking a random List element is a very basic operation but not so obvious to implement. In this article, we’ll show the most efficient way […]
1. Overview Creating a multidimensional ArrayList often comes up during programming. In many cases, there is a need to create a two-dimensional ArrayList or a three-dimensional ArrayList. In this tutorial, we’ll discuss […]
1. Overview This quick tutorial will show how to make an ArrayList immutable with the core JDK, with Guava and finally with Apache Commons Collections 4. This article is […]
1. Overview In this article, we’re going to take a look at ArrayList class from the Java Collections Framework. We’ll discuss its properties, common use cases, as […]
1. Introduction LinkedList is a doubly-linked list implementation of the List and Deque interfaces. It implements all optional list operations and permits all elements (including null). 2. Features Below you can […]
1. Introduction Concurrency in Java is one of the most complex and advanced topics brought up during technical interviews. This article provides answers to some […]
1. Introduction The Dining Philosophers problem is one of the classic problems used to describe synchronization issues in a multi-threaded environment and illustrate techniques for solving […]
1. Introduction It is relatively common for Java programs to add a delay or pause in their operation. This can be useful for task pacing […]
1. Introduction In this tutorial, we’ll compare CyclicBarrier and CountDownLatch and try to understand the similarities and differences between the two. 2. What Are These? When it comes to […]
1. Overview Generating random values is a very common task. This is why Java provides the java.util.Random class. However, this class doesn’t perform well in a multi-threaded […]
1. Introduction CyclicBarriers are synchronization constructs that were introduced with Java 5 as a part of the java.util.concurrent package. In this article, we’ll explore this implementation in a […]
1. Introduction In this article, we’ll give a guide to the CountDownLatch class and demonstrate how it can be used in a few practical examples. Essentially, by […]