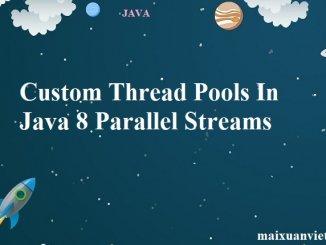
Custom Thread Pools In Java 8 Parallel Streams
1. Overview Java 8 introduced the concept of Streams as an efficient way of carrying out bulk operations on data. And parallel Streams can be obtained in environments […]
1. Overview Java 8 introduced the concept of Streams as an efficient way of carrying out bulk operations on data. And parallel Streams can be obtained in environments […]
1. Overview The fork/join framework was presented in Java 7. It provides tools to help speed up parallel processing by attempting to use all available […]
1. Overview ExecutorService is a JDK API that simplifies running tasks in asynchronous mode. Generally speaking, ExecutorService automatically provides a pool of threads and an API for assigning […]
1. Overview In this short article, we’ll have a look at daemon threads in Java and see what can they be used for. We’ll also […]
1. Overview In this tutorial, we’ll see different ways to implement a mutex in Java. 2. Mutex In a multithreaded application, two or more threads may need to […]
1. Overview In this tutorial, we’ll discuss the different join() methods in the Thread class. We’ll go into the details of these methods and some example code. Like the wait() and notify() methods, join() is […]
1. Overview In this short article, we’ll have a look at the standard sleep() and wait() methods in core Java, and understand the differences and similarities between them. 2. […]
1. Overview Since the early days of Java, multithreading has been a major aspect of the language. Runnable is the core interface provided for representing multi-threaded tasks […]
1. Overview In this tutorial, we’ll look at one of the most fundamental mechanisms in Java — thread synchronization. We’ll first discuss some essential concurrency-related […]
1. Overview This tutorial is a look at thread pools in Java. We’ll start with the different implementations in the standard Java library and then […]
1. Introduction In this article, we’ll discuss in detail a core concept in Java – the lifecycle of a thread. We’ll use a quick illustrated […]
1. Overview In this article, we will be looking at the ThreadLocal construct from the java.lang package. This gives us the ability to store data individually for the current […]
1. Overview In the absence of necessary synchronizations, the compiler, runtime, or processors may apply all sorts of optimizations. Even though these optimizations are beneficial […]
1. Overview This quick article will be an intro to using the synchronized block in Java. Simply put, in a multi-threaded environment, a race condition occurs when two or […]
1. Overview In this tutorial, we’ll look at different approaches to number formatting in Java and how to implement them. 2. Basic Number Formatting with String#format […]
1. Overview There are many ways to check if a String contains a substring. In this article, we’ll be looking for substrings within a String while focusing on case-insensitive […]
1. Overview In this short tutorial, we’ll discuss different ways to compare two Long instances. We emphasize the problems that arise when using the reference comparison operator […]
1. Introduction In this tutorial, we’ll look at the overflow and underflow of numerical data types in Java. We won’t dive deeper into the more […]
1. Overview Java exceptions fall into two main categories: checked exceptions and unchecked exceptions. In this article, we’ll provide some code samples on how to use them. […]
1. Overview In this short tutorial, we’re going to learn about the Java XOR operator. We’ll go through a bit of theory about XOR operations, and then we’ll see […]