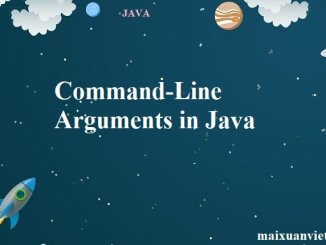
Command-Line Arguments in Java
1. Introduction It’s quite common to run applications from the command-line using arguments. Especially on the server-side. Usually, we don’t want the application to do the […]
1. Introduction It’s quite common to run applications from the command-line using arguments. Especially on the server-side. Usually, we don’t want the application to do the […]
1. Introduction A copy constructor in a Java class is a constructor that creates an object using another object of the same Java class. That’s helpful when we […]
1. Introduction In this tutorial, we’ll focus on how to compare dates using the Java 8 Date/Time API. We’ll dive into different methods to check whether […]
1. Overview In this quick tutorial, we’ll illustrate multiple ways of converting time into Unix-epoch milliseconds in Java. More specifically, we’ll use: Core Java’s java.util.Date and Calendar Java 8’s […]
1. Introduction In this tutorial, we’ll consider anonymous classes in Java. We’ll describe how we can declare and create instances of them. We’ll also briefly […]
1. Overview Every class in Java is a child of the Object class either directly or indirectly. And since the Object class contains a toString() method, we can call toString() on any instance and […]
1. Overview In this tutorial, we’ll learn what the switch statement is and how to use it. The switch statement allows us to replace several nested if-else constructs and thus improve […]
1. Overview In this short tutorial, we’re going to show what the modulo operator is, and how we can use it with Java for some […]
1. Introduction In Java, Strings are immutable. An obvious question that is quite prevalent in interviews is “Why Strings are designed as immutable in Java?” […]
1. Overview In this tutorial, we’ll learn what makes an object immutable, how to achieve immutability in Java, and what advantages come with doing so. […]
1. Overview In this tutorial, we’ll cover how to create a custom exception in Java. We’ll show how user-defined exceptions are implemented and used for both […]
1. Overview In this tutorial, we’ll go through the basics of exception handling in Java as well as some of its gotchas. 2. First Principles […]
1. Overview While inheritance enables us to reuse existing code, sometimes we do need to set limitations on extensibility for various reasons; the final keyword allows us to do […]
1. Introduction Regular expressions are a powerful tool for matching various kinds of patterns when used appropriately. In this article, we’ll use java.util.regex package to determine whether […]
1. Overview In this quick tutorial, we’re going to take a look at two new classes for working with dates introduced in Java 8: Period and Duration. Both […]
1. Introduction When dealing with exceptions in Java, we’re frequently logging or simply displaying stack traces. However, sometimes, we don’t want just to print the […]
1. Overview StackOverflowError can be annoying for Java developers, as it’s one of the most common runtime errors we can encounter. In this article, we’ll see […]
1. Overview Enum in Java is a datatype that helps us assign a predefined set of constants to a variable. In this quick article, we’ll see […]
1. Overview UUID (Universally Unique Identifier), also known as GUID (Globally Unique Identifier) represents a 128-bit long value that is unique for all practical purposes. The standard representation […]
1. Overview In this short tutorial, we’ll learn how to round a number to n decimal places in Java. 2. Decimal Numbers in Java Java provides two […]