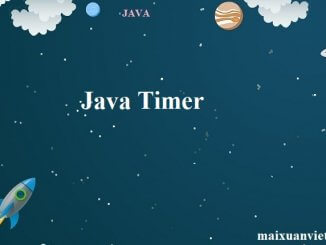
Java Timer
1. Timer – the Basics Timer and TimerTask are java util classes used to schedule tasks in a background thread. In a few words – TimerTask is the task to […]
1. Timer – the Basics Timer and TimerTask are java util classes used to schedule tasks in a background thread. In a few words – TimerTask is the task to […]
1. Generate an Unbounded Long Let’s start with generating a Long: 2. Generate a Long Within a Range 2.1. Random Long With Plain Java Next […]
1. Overview In this tutorial, we’re going to take a first look at the Lambda support in Java 8 – specifically at how to leverage it […]
1. Introduction The binary number system uses 0s and 1s to represent numbers. Computers use binary numbers to store and perform operations on any data. […]
1. Introduction Comparing objects is an essential feature of object-oriented programming languages. In this tutorial, we’re going look at some of the features of the […]
1. Introduction In Java’s if-else statements we can take a certain action when an expression is true, and an alternative when it is false. In this tutorial, we’ll learn […]
1. Overview In the most basic sense, a program is a list of instructions. Control structures are programming blocks that can change the path we take […]
1. Introduction In this quick guide, we’ll discuss the term “concrete class” in Java. First, we’ll define the term. Then, we’ll see how it’s different from […]
1. Overview In this tutorial, we’ll focus on a core aspect of the Java language – the finalize method provided by the root Object class. Simply put, this is […]
1. Overview The Java type system is made up of two kinds of types: primitives and references. We covered primitive conversions in this article, and we’ll […]
1. Overview As the name suggests, wrapper classes are objects encapsulating primitive Java types. Each Java primitive has a corresponding wrapper: boolean, byte, short, char, int, […]
1. Overview In the absence of necessary synchronizations, the compiler, runtime, or processors may apply all sorts of optimizations. Even though these optimizations are beneficial […]
1. Overview Inheritance and composition — along with abstraction, encapsulation, and polymorphism — are cornerstones of object-oriented programming (OOP). In this tutorial, we’ll cover the basics of inheritance […]
1. Introduction The Java assert keyword allows developers to quickly verify certain assumptions or state of a program. In this article, we’ll take a look at how to […]
1. Introduction to Class Loaders Class loaders are responsible for loading Java classes during runtime dynamically to the JVM (Java Virtual Machine). Also, they are part of […]
1. Overview Java 9 introduces a new level of abstraction above packages, formally known as the Java Platform Module System (JPMS), or “Modules” for short. […]
1. Overview Support for try-with-resources — introduced in Java 7 — allows us to declare resources to be used in a try block with the assurance that the resources […]
1. Overview Debugging reactive streams is probably one of the main challenges we’ll have to face once we start using these data structures. And having in mind […]
1. Introduction In this tutorial, we’ll explore several libraries for reading an HTTP response body as a string in Java. Since the first versions, Java […]
1. Overview This tutorial focuses on the basic principles and mechanics of testing a REST API with live Integration Tests (with a JSON payload). The main goal […]