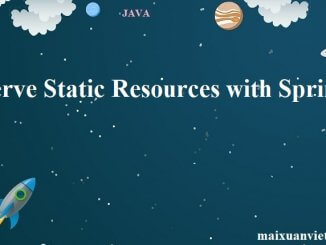
Serve Static Resources with Spring
1. Overview This article explores how to serve static resources with Spring – using both XML and Java configuration. 2. Using Spring Boot Spring Boot comes with […]
1. Overview This article explores how to serve static resources with Spring – using both XML and Java configuration. 2. Using Spring Boot Spring Boot comes with […]
1. Introduction In this article we’ll focus on a core concept in Spring MVC – Controllers. 2. Overview Let’s start by taking a step back […]
1. Introduction Thymeleaf is a Java template engine for processing and creating HTML, XML, JavaScript, CSS, and text. In this article, we will discuss how to use […]
1. Overview Spring 4.3. introduced some very cool method-level composed annotations to smooth out the handling @RequestMapping in typical Spring MVC projects. In this article, we will learn how […]
1. Introduction In this quick tutorial, we provide a concise overview of the Spring @RequestBody and @ResponseBody annotations. 2. @RequestBody Simply put, the @RequestBody annotation maps the HttpRequest body to a transfer or domain object, […]
1. Overview In this brief tutorial, we’ll discuss the difference between @Controller and @RestController annotations in Spring MVC. We can use the first annotation for traditional Spring controllers, and […]
1. Overview In this quick tutorial, we’ll explore Spring’s @RequestParam annotation and its attributes. Simply put, we can use @RequestParam to extract query parameters, form parameters, and even files from […]
1. Overview In this tutorial, we’ll focus on one of the main annotations in Spring MVC: @RequestMapping. Simply put, the annotation is used to map web requests […]
1. Overview In this tutorial, we’ll secure a REST API with OAuth and consume it from a simple Angular client. The application we’re going to […]
1. Overview In this tutorial, we’ll continue exploring the OAuth2 Authorization Code flow that we started putting together in our previous article and we’ll focus on how to […]
1. Overview This tutorial will continue to explore some of the core features of Spring Data MongoDB – the @DBRef annotation and life-cycle events. 2. @DBRef The mapping […]
1. Introduction MyBatis is one of the most commonly used open-source frameworks for implementing SQL databases access in Java applications. In this quick tutorial, we’ll present […]
1. Introduction In this quick tutorial, we’re going to cover various ways of creating LIKE queries in Spring JPA Repositories. We’ll start by looking at the […]
1. Overview This tutorial will focus on introducing Spring Data JPA into a Spring project, and fully configuring the persistence layer. For a step by step introduction […]
1. Overview This tutorial shows how to set up Spring with JPA, using Hibernate as a persistence provider. For a step-by-step introduction to setting up the […]
1. Overview Spring JDBC and JPA provide abstractions over native JDBC APIs, allowing developers to do away with native SQL queries. However, we often need to see those auto-generated […]
1. Overview This article will focus on simplifying the DAO layer by using a single, generified Data Access Object for all entities in the system, which will […]
1. Overview This article will show how to implement the DAO with Spring and JPA. For the core JPA configuration, see the article about JPA with Spring. 2. […]
1. Overview This article will show how to implement the DAO with Spring and Hibernate. For the core Hibernate configuration, check out the previous Hibernate 5 with […]
1. Overview Spring’s @Transactional annotation provides a nice declarative API to mark transactional boundaries. Behind the scenes, an aspect takes care of creating and maintaining transactions as […]