In this program, you will learn to convert decimal number to binary using recursive function.
o understand this example, you should have the knowledge of the following Python programming topics:
Decimal number is converted into binary by dividing the number successively by 2 and printing the remainder in reverse order.
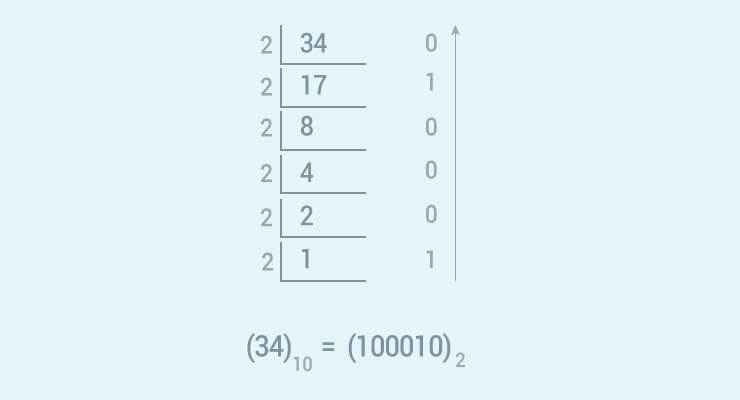
Source Code
# Function to print binary number using recursion def convertToBinary(n): if n > 1: convertToBinary(n//2) print(n % 2,end = '') # decimal number dec = 34 convertToBinary(dec) print()
Output
100010
You can change the variable dec in the above program and run it to test out for other values.
This program works only for whole numbers. It doesn’t work for real numbers having fractional values such as: 25.5, 45.64 and so on. We encourage you to create Python program that converts decimal numbers to binary for all real numbers on your own.
Related posts:
Python String islower()
Python len()
Python sum()
Python callable()
Python String isalnum()
Node.js vs Python for Backend Development
Python Data Types
Python String expandtabs()
Python Program to Find All File with .txt Extension Present Inside a Directory
Python help()
Python Exception Handling Using try, except and finally statement
Python Program to Transpose a Matrix
Python setattr()
Binary Exponentiation
Python Program to Get File Creation and Modification Date
Python Set symmetric_difference()
Python Program to Trim Whitespace From a String
Python Set isdisjoint()
Python while Loop
Python Modules
Python bool()
JavaScript Recursion and stack
Python Program to Check If a List is Empty
Python Program to Merge Two Dictionaries
Python Program to Display the multiplication Table
Python String zfill()
Python Set pop()
Python Program to Print the Fibonacci sequence
Intelligent Projects Using Python - Santanu Pattanayak
Python String center()
Python Dictionary clear()
Python Program to Find HCF or GCD