Table of Contents
In this tutorial, we will learn about the Python Set union() method with the help of example.
The Python set union()
method returns a new set with distinct elements from all the sets.
Example
A = {2, 3, 5} B = {1, 3, 5} # compute union between A and B print('A U B = ', A.union(B)) # Output: A U B = {1, 2, 3, 5}
1. Syntax of Set union()
The syntax of union()
is:
A.union(*other_sets)
Note: * is not part of the syntax. It is used to indicate that the method can take 0 or more arguments.
2. Return Value from union()
- The
union()
method returns a new set with elements from the set and all other sets (passed as an argument). - If the argument is not passed to
union()
, it returns a shallow copy of the set.
3. Example 1: Python Set union()
A = {'a', 'c', 'd'} B = {'c', 'd', 2 } C = {1, 2, 3} print('A U B =', A.union(B)) print('B U C =', B.union(C)) print('A U B U C =', A.union(B, C)) print('A.union() =', A.union())
Output
A U B = {2, 'a', 'd', 'c'} B U C = {1, 2, 3, 'd', 'c'} A U B U C = {1, 2, 3, 'a', 'd', 'c'} A.union() = {'a', 'd', 'c'}
4. Working of Set Union
The union of two or more sets is the set of all distinct elements present in all the sets. For example:
A = {1, 2} B = {2, 3, 4} C = {5} Then, A∪B = B∪A = {1, 2, 3, 4} A∪C = C∪A = {1, 2, 5} B∪C = C∪B = {2, 3, 4, 5} A∪B∪C = {1, 2, 3, 4, 5}
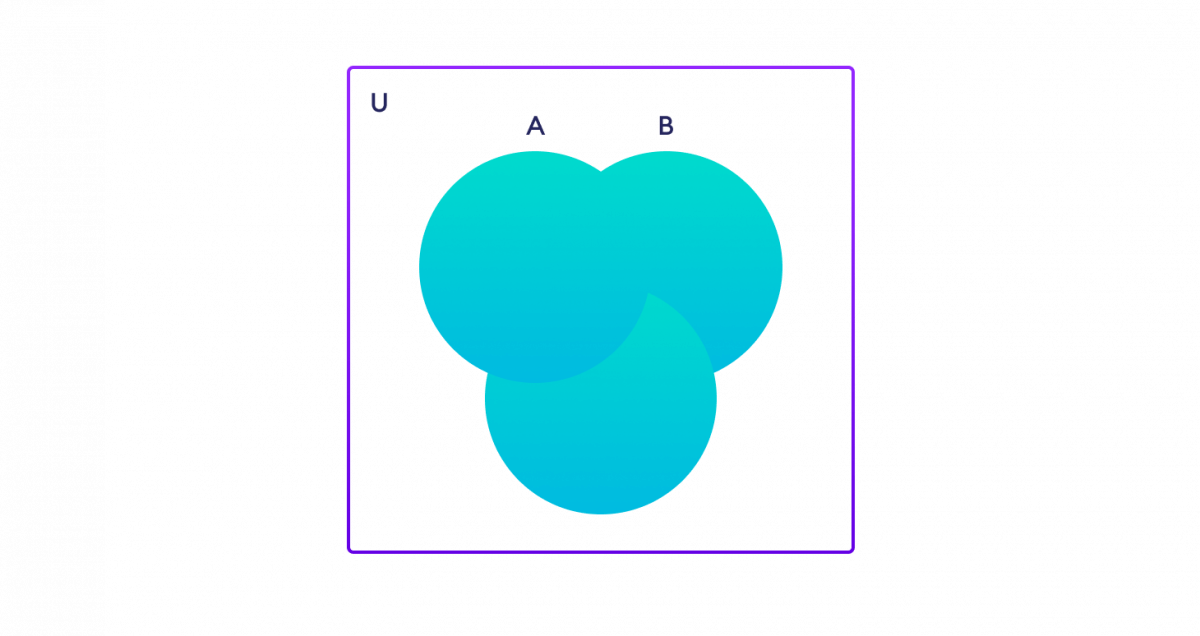
5. Example 2: Set Union Using the | Operator
You can also find the union of sets using the |
operator.
A = {'a', 'c', 'd'} B = {'c', 'd', 2 } C = {1, 2, 3} print('A U B =', A| B) print('B U C =', B | C) print('A U B U C =', A | B | C)
Output
A U B = {2, 'a', 'c', 'd'} B U C = {1, 2, 3, 'c', 'd'} A U B U C = {1, 2, 3, 'a', 'c', 'd'}
Related posts:
Python Set issubset()
Python Global Keyword
Python String count()
Deep Learning with Python - A Hands-on Introduction - Nikhil Ketkar
Python Program to Delete an Element From a Dictionary
Python complex()
Python Dictionary popitem()
Python Machine Learning Second Edition - Sebastian Raschka & Vahid Mirjalili
Python bin()
Python @property decorator
Python Data Types
Python String istitle()
Python Program to Differentiate Between del, remove, and pop on a List
Python Dictionary items()
Python bytes()
Python Program to Check Prime Number
Python Program to Check If a String Is a Number (Float)
Python Tuple index()
Python for Programmers with introductory AI case studies - Paul Deitel & Harvey Deitel
Python timestamp to datetime and vice-versa
Python Closures
Python len()
Python String isnumeric()
Python Input, Output and Import
Python Program to Print Output Without a Newline
Python Program to Convert Celsius To Fahrenheit
Python Program to Find the Factors of a Number
Python str()
Python Program to Generate a Random Number
Python Dictionary setdefault()
Python List index()
Converting Between an Array and a Set in Java