Table of Contents
The rfind() method returns the highest index of the substring (if found). If not found, it returns -1.
The syntax of rfind()
is:
str.rfind(sub[, start[, end]] )
1. rfind() Parameters
rfind()
method takes a maximum of three parameters:
- sub – It’s the substring to be searched in the str string.
- start and end (optional) – substring is searched within
str[start:end]
2. Return Value from rfind()
rfind()
method returns an integer value.
- If substring exists inside the string, it returns the highest index where substring is found.
- If substring doesn’t exist inside the string, it returns -1.
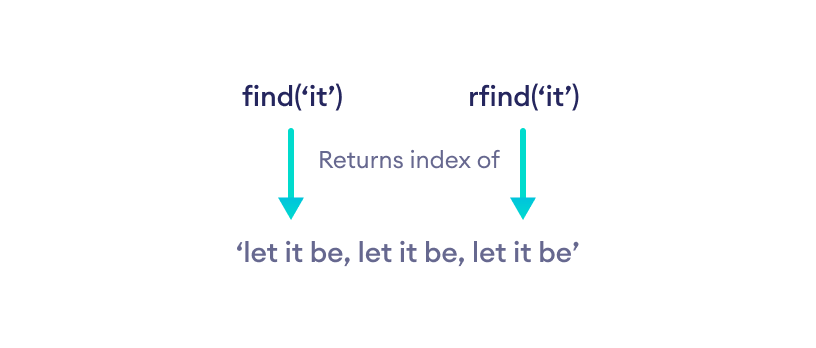
3. Example 1: rfind() With No start and end Argument
quote = 'Let it be, let it be, let it be' result = quote.rfind('let it') print("Substring 'let it':", result) result = quote.rfind('small') print("Substring 'small ':", result) result = quote.rfind('be,') if (result != -1): print("Highest index where 'be,' occurs:", result) else: print("Doesn't contain substring")
Output
Substring 'let it': 22 Substring 'small ': -1 Highest index where 'be,' occurs: 18
4. Example 2: rfind() With start and end Arguments
quote = 'Do small things with great love' # Substring is searched in 'hings with great love' print(quote.rfind('things', 10)) # Substring is searched in ' small things with great love' print(quote.rfind('t', 2)) # Substring is searched in 'hings with great lov' print(quote.rfind('o small ', 10, -1)) # Substring is searched in 'll things with' print(quote.rfind('th', 6, 20))
Output
-1 25 -1 18
Related posts:
Python Program to Check if a Number is Odd or Even
Python all()
Python range()
Python id()
Check if a String is a Palindrome in Java
Python Program to Get a Substring of a String
Java InputStream to String
Introduction to Machine Learning with Python - Andreas C.Muller & Sarah Guido
Python isinstance()
Python Set difference()
Python Set difference_update()
Python if...else Statement
Python Program to Make a Simple Calculator
Python Global Keyword
Python Program to Find LCM
Learning scikit-learn Machine Learning in Python - Raul Garreta & Guillermo Moncecchi
Python Set clear()
Python sorted()
Introduction to Scientific Programming with Python - Joakim Sundnes
Python Set update()
Python List reverse()
Python String isupper()
Natural Language Processing with Python - Steven Bird & Ewan Klein & Edward Loper
Python Program to Find All File with .txt Extension Present Inside a Directory
Python Set discard()
Python Set intersection()
Building Machine Learning Systems with Python - Willi Richert & Luis Pedro Coelho
Python Program to Transpose a Matrix
Python Program to Extract Extension From the File Name
Python Program to Get the File Name From the File Path
Python Program to Print the Fibonacci sequence
Python Program to Sort Words in Alphabetic Order