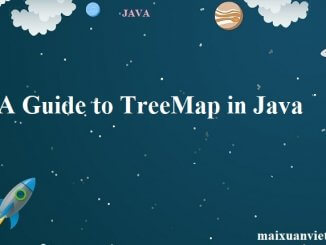
A Guide to TreeMap in Java
1. Overview In this article, we are going to explore TreeMap implementation of Map interface from Java Collections Framework(JCF). TreeMap is a map implementation that keeps its entries sorted according […]
1. Overview In this article, we are going to explore TreeMap implementation of Map interface from Java Collections Framework(JCF). TreeMap is a map implementation that keeps its entries sorted according […]
1. Overview In this article, we’ll see how to use HashMap in Java, and we’ll look at how it works internally. A class very similar to HashMap is Hashtable. Please […]
1. Overview In this article, we’ll dive into HashSet. It’s one of the most popular Set implementations as well as an integral part of the Java Collections Framework. 2. […]
1. Overview In this article, we’ll have a look at an integral part of the Java Collections Framework and one of the most popular Set implementations – the TreeSet. […]
1. Overview In this quick article, we’ll be looking at the CopyOnWriteArrayList from the java.util.concurrent package. This is a very useful construct in the multi-threaded programs – when we […]
1. Overview In this article, we’re going to take a look at ArrayList class from the Java Collections Framework. We’ll discuss its properties, common use cases, as […]
1. Introduction LinkedList is a doubly-linked list implementation of the List and Deque interfaces. It implements all optional list operations and permits all elements (including null). 2. Features Below you can […]
1. Overview Generating random values is a very common task. This is why Java provides the java.util.Random class. However, this class doesn’t perform well in a multi-threaded […]
1. Introduction This tutorial is a guide to the functionality and use cases of the CompletableFuture class that was introduced as a Java 8 Concurrency API improvement. […]
1. Overview Simply put, a lock is a more flexible and sophisticated thread synchronization mechanism than the standard synchronized block. The Lock interface has been around since Java 1.5. […]
1. Introduction In this article, we’ll give a guide to the CountDownLatch class and demonstrate how it can be used in a few practical examples. Essentially, by […]
1. Overview The fork/join framework was presented in Java 7. It provides tools to help speed up parallel processing by attempting to use all available […]
1. Overview ExecutorService is a JDK API that simplifies running tasks in asynchronous mode. Generally speaking, ExecutorService automatically provides a pool of threads and an API for assigning […]
1. Overview In this article, we are going to learn about Future. An interface that’s been around since Java 1.5 and can be quite useful when […]
1. Overview In the absence of necessary synchronizations, the compiler, runtime, or processors may apply all sorts of optimizations. Even though these optimizations are beneficial […]
1. Overview This quick article will be an intro to using the synchronized block in Java. Simply put, in a multi-threaded environment, a race condition occurs when two or […]
1. Overview UUID (Universally Unique Identifier), also known as GUID (Globally Unique Identifier) represents a 128-bit long value that is unique for all practical purposes. The standard representation […]
1. Overview In this tutorial, we’ll focus on a core aspect of the Java language – the finalize method provided by the root Object class. Simply put, this is […]
1. Overview In the absence of necessary synchronizations, the compiler, runtime, or processors may apply all sorts of optimizations. Even though these optimizations are beneficial […]
1. Overview Java 9 introduces a new level of abstraction above packages, formally known as the Java Platform Module System (JPMS), or “Modules” for short. […]