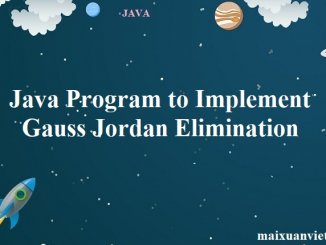
Java Program to Implement Gauss Jordan Elimination
This is java program to find the solution to the linear equations of any number of variables using the method of Gauss-Jordan algorithm. Here is […]
This is java program to find the solution to the linear equations of any number of variables using the method of Gauss-Jordan algorithm. Here is […]
This is the java implementation of classic Coppersmith-Freivalds’ algorithm to check whether the multiplication of matrix A and B equals the given matrix C. It […]
This is a java program to LU Decomposition of a given matrix. LU decomposition is the process of reducing single matrix into 2 matrices such […]
This is a java program to encrypt a matrix using a key. The key is hidden and kept secret and inverse copy of the key […]
This is the java program to find the inverse of square invertible matrix. The matrix is invertible if its determinant is non zero. Here is […]
This is java program to solve the system of linear equations. This can be done by first representing equations(vectors) to matrix form, then finding the […]
1. Java String.String() String objects can be created by either using literals: or by calling one of the constructors: If we use the String literal, it’ll try to reuse […]
1. Overview In this tutorial, we’ll look at how to convert an InputStream to a String. We’ll start by using plain Java, including Java8/9 solutions, then look into […]
1. Introduction Converting char to String instances is a very common operation. In this article, we will show multiple ways of tackling this situation. 2. String.valueOf() The String class has a […]
1. Introduction Converting a String to an int or Integer is a very common operation in Java. In this article, we will show multiple ways of dealing with this issue. There […]
1. Overview When dealing with Strings in Java, sometimes we need to encode them into a specific charset. This tutorial is a practical guide showing different […]
1. Introduction We frequently need to convert between String and byte array in Java. In this tutorial, we’ll examine these operations in detail. First, we’ll look at various ways […]
1. Overview In this tutorial, we’ll take a look at different ways to convert a byte array to a hexadecimal String, and vice versa. We’ll also understand […]
1. Introduction Oftentimes while operating upon Strings, we need to figure out whether a String is a valid number or not. In this tutorial, we’ll explore multiple ways to […]
1. Overview In this quick article, we’re going to do some simple conversions between the Hex and ASCII formats. In a typical use case, the […]
1. Introduction In this quick article, we’ll have a look at how to convert a List of elements to a String. This might be useful in certain scenarios […]
1. Introduction When dealing with exceptions in Java, we’re frequently logging or simply displaying stack traces. However, sometimes, we don’t want just to print the […]
1. Introduction In this short article, we will see how to convert a String into an enum in Java quickly. 2. Setup We are dealing […]
1. Overview In this quick article, we’ll explore some simple conversions of String objects to different data types supported in Java. 2. Converting String to int or Integer If we need to […]
1. Overview In this short article, we’re going to look at similarities and differences between StringBuilder and StringBuffer in Java. Simply put, StringBuilder was introduced in Java 1.5 as a replacement […]