Table of Contents
1. Overview
The symmetric_difference_update() method finds the symmetric difference of two sets and updates the set calling it.
The symmetric difference of two sets A and B is the set of elements that are in either A or B, but not in their intersection.
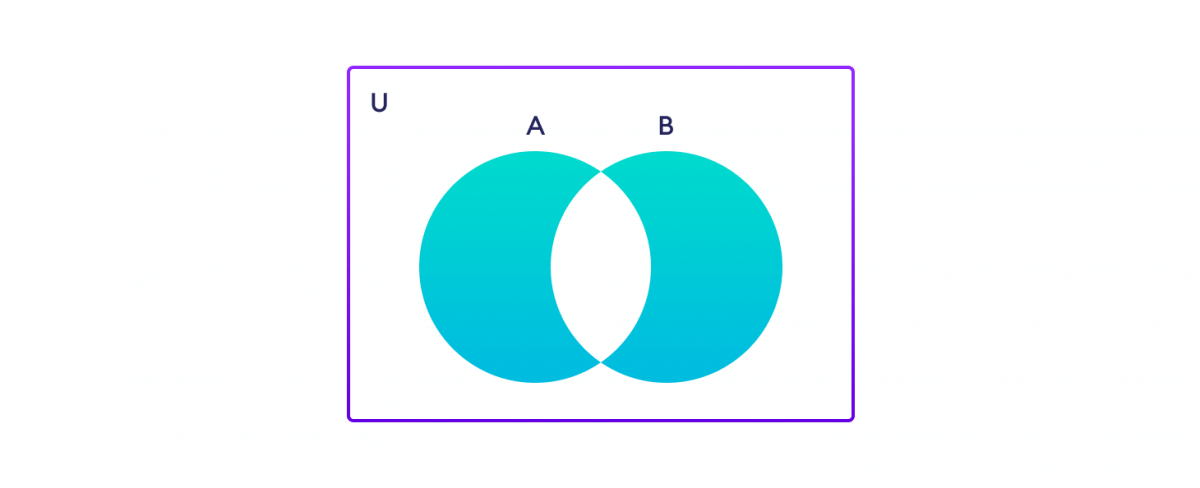
The syntax of symmetric_difference_update()
is:
A.symmetric_difference_update(B)
2. Return Value from symmetric_difference_update()
- The
symmetric_difference_update()
returnsNone
(returns nothing). Rather, it updates the set calling it.
3. Example: Working of symmetric_difference_update()
A = {'a', 'c', 'd'} B = {'c', 'd', 'e' } result = A.symmetric_difference_update(B) print('A =', A) print('B =', B) print('result =', result)
Output
A = {'a', 'e'} B = {'d', 'c', 'e'} result = None
Here, the set A is updated with the symmetric difference of set A and B. However, the set B
is unchanged.
Recommended Reading: Python Set symmetric_difference()
Related posts:
Python Set isdisjoint()
Python String rpartition()
Python filter()
Python open()
Python Errors and Built-in Exceptions
Python Type Conversion and Type Casting
Python Machine Learning Cookbook - Practical solutions from preprocessing to Deep Learning - Chris A...
Python Program to Find Armstrong Number in an Interval
Python Multiple Inheritance
Python Set intersection()
Python String startswith()
Python enumerate()
Deep Learning with Python - Francois Cholletf
Python Program to Measure the Elapsed Time in Python
Python Program to Solve Quadratic Equation
Python Program to Catch Multiple Exceptions in One Line
Node.js vs Python for Backend Development
Python Program to Convert Decimal to Binary Using Recursion
Python Program to Check if a Key is Already Present in a Dictionary
Python Program to Iterate Over Dictionaries Using for Loop
Python String islower()
Python delattr()
Python pow()
Python len()
Python Program to Calculate the Area of a Triangle
Python Program to Check the File Size
Python String rsplit()
Python Program to Parse a String to a Float or Int
Python Operator Overloading
Python String isdecimal()
Python Statement, Indentation and Comments
Python type()