You are given a set of size m with integer elements between 0 and 2n−1 inclusive. Let’s build an undirected graph on these integers in the following way: connect two integers x and y with an edge if and only if x&y=0. Here & is the bitwise AND operation. Count the number of connected components in that graph.
Input
In the first line of input there are two integers n and m (0≤n≤22, 1≤m≤2n).
In the second line there are m integers a1,a2,…,am (0≤ai<2n) — the elements of the set. All ai are distinct.
Output
Print the number of connected components.
Examples
input
2 3
1 2 3
output
2
input
5 5
5 19 10 20 12
output
2
Note
Graph from first sample:
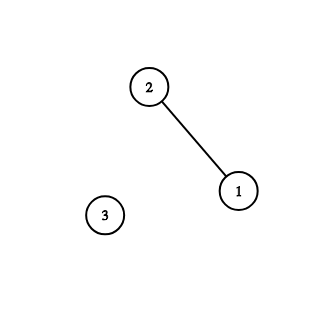
Graph from second sample:
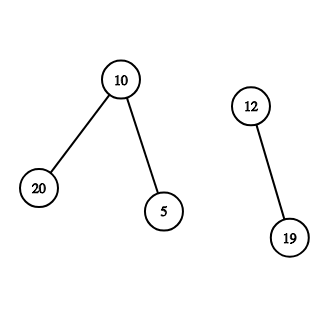
Solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | #include <bits/stdc++.h> using namespace std; int main() { ios::sync_with_stdio( false ); cin.tie(0); int n, m; cin >> n >> m; vector< int > was(1 << n, 1); for ( int i = 0; i < m; i++) { int x; cin >> x; was[x] = 0; } vector< int > used(1 << n, 0); vector< int > que(1 << n); vector< int > inner(1 << n); int ans = 0; for ( int start = 0; start < (1 << n); start++) { if (was[start]) { continue ; } ans++; was[start] = 1; que[0] = start; int qs = 1; for ( int q = 0; q < qs; q++) { int u = (1 << n) - 1 - que[q]; if (!used[u]) { used[u] = 1; inner[0] = u; int is = 1; for ( int i = 0; i < is; i++) { int z = inner[i]; if (!was[z]) { was[z] = 1; que[qs++] = z; } for ( int bit = 0; bit < n; bit++) { if ((z & (1 << bit)) && !used[z ^ (1 << bit)]) { used[z ^ (1 << bit)] = 1; inner[is++] = z ^ (1 << bit); } } } } } } cout << ans << '\n' ; return 0; } |
Related posts:
Golden System
Teams
Lingua Romana
Lowest Common Ancestor
Minimum stack / Minimum queue
Résumé Review
Alex and a TV Show
Robbers' watch
Attack on Red Kingdom
Irreducible Anagrams
Rabin-Karp Algorithm for string matching
Giải Thuật Và Lập Trình - Lê Minh Hoàng
Weird Game
Born This Way
Context Advertising
Bingo!
Jongmah
Largest Submatrix 3
Information Graph
Earth Wind and Fire
New Year and Rating
Alternating Current
Submask Enumeration
GCD Table
Robot Sequence
New Year and Old Property
Playing with Permutations
Field expansion
Bears and Juice
Cut the pie
Salazar Slytherin's Locket
Recommendations