Due to the recent popularity of the Deep learning new countries are starting to look like Neural Networks. That is, the countries are being built deep with many layers, each layer possibly having many cities. They also have one entry, and one exit point.
There are exactly L layers, each having N cities. Let us look at the two adjacent layers L 1 and L 2. Each city from the layer L 1 is connected to each city from the layer L 2 with the traveling cost c ij for , and each pair of adjacent layers has the same cost in between their cities as any other pair (they just stacked the same layers, as usual). Also, the traveling costs to each city from the layer L 2 are same for all cities in the L 1, that is c ij is the same for
, and fixed j.
Doctor G. needs to speed up his computations for this country so he asks you to find the number of paths he can take from entry to exit point such that his traveling cost is divisible by given number M.
Input
The first line of input contains N (1 ≤ N ≤ 106), L (2 ≤ L ≤ 105) and M (2 ≤ M ≤ 100), the number of cities in each layer, the number of layers and the number that travelling cost should be divisible by, respectively.
Second, third and fourth line contain N integers each denoting costs 0 ≤ cost ≤ M from entry point to the first layer, costs between adjacent layers as described above, and costs from the last layer to the exit point.
Output
Output a single integer, the number of paths Doctor G. can take which have total cost divisible by M, modulo 109 + 7.
Example
input
2 3 13
4 6
2 1
3 4
output
2
Note
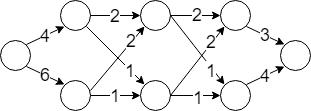
This is a country with 3 layers, each layer having 2 cities. Paths , and
are the only paths having total cost divisible by 13. Notice that input edges for layer cities have the same cost, and that they are same for all layers.
Solution:
#include <bits/stdc++.h> using namespace std; const int md = 1000000007; inline void add(int &a, int b) { a += b; if (a >= md) { a -= md; } } inline int mul(int a, int b) { return (long long) a * b % md; } inline int power(int a, int b) { int res = 1; while (b > 0) { if (b & 1) { res = mul(res, a); } a = mul(a, a); b >>= 1; } return res; } inline int inv(int x) { return power(x, md - 2); } const int N = 105; struct matrix { int a[N][N]; }; int m; matrix multiply(matrix a, matrix b) { matrix c; for (int i = 0; i < m; i++) { for (int j = 0; j < m; j++) { c.a[i][j] = 0; for (int k = 0; k < m; k++) { add(c.a[i][j], mul(a.a[i][k], b.a[k][j])); } } } return c; } matrix power(matrix a, int b) { matrix c; for (int i = 0; i < m; i++) { c.a[i][i] = 1; } while (b > 0) { if (b & 1) { c = multiply(c, a); } a = multiply(a, a); b >>= 1; } return c; } int last[1234567]; int not_last[1234567]; int main() { int n, l; scanf("%d %d %d", &n, &l, &m); matrix a, b; for (int i = 0; i < n; i++) { int foo; scanf("%d", &foo); a.a[0][foo % m]++; } for (int i = 0; i < n; i++) { scanf("%d", not_last + i); b.a[0][not_last[i] % m]++; } for (int i = 0; i < n; i++) { scanf("%d", last + i); } for (int i = 1; i < m; i++) { for (int j = 0; j < m; j++) { a.a[i][(i + j) % m] = a.a[0][j]; b.a[i][(i + j) % m] = b.a[0][j]; } } matrix d = multiply(power(b, l - 2), a); int ans = 0; for (int i = 0; i < n; i++) { int z = (last[i] + not_last[i]) % m; add(ans, d.a[0][(m - z) % m]); } printf("%d\n", ans); return 0; }