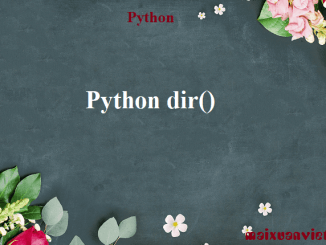
Python dir()
The dir() method tries to return a list of valid attributes of the object. The syntax of dir() is: 1. dir() Parameters dir() takes maximum of one object. […]
The dir() method tries to return a list of valid attributes of the object. The syntax of dir() is: 1. dir() Parameters dir() takes maximum of one object. […]
The dict() constructor creates a dictionary in Python. Different forms of dict() constructors are: Note: **kwarg let you take an arbitrary number of keyword arguments. A keyword argument is […]
The delattr() deletes an attribute from the object (if the object allows it). The syntax of delattr() is: 1. delattr() Parameters delattr() takes two parameters: object – the object […]
The complex() method returns a complex number when real and imaginary parts are provided, or it converts a string to a complex number. The syntax […]
In this tutorial, we will learn about the Python classmethod() function with the help of examples. The classmethod() method returns a class method for the given function. […]
The compile() method returns a Python code object from the source (normal string, a byte string, or an AST object). The syntax of compile() is: compile() method is […]
The chr() method returns a character (a string) from an integer (represents unicode code point of the character). The syntax of chr() is: 1. chr() Parameters chr() method […]
In this tutorial, we will learn about the Python bytes() method with the help of examples. The bytes() method returns an immutable bytes object initialized with the […]
The callable() method returns True if the object passed appears callable. If not, it returns False. The syntax of callable() is: 1. callable() Parameters callable() method takes a […]
In this tutorial, we will learn about the Python bytearray() method with the help of examples. The bytearray() method returns a bytearray object which is an array […]
The bool() function converts a value to Boolean (True or False) using the standard truth testing procedure. The syntax of bool() is: 1. bool() parameters It’s not […]
The bin() method converts and returns the binary equivalent string of a given integer. If the parameter isn’t an integer, it has to implement __index__() […]
The ascii() method returns a string containing a printable representation of an object. It escapes the non-ASCII characters in the string using \x, \u or […]
In this tutorial, we will learn about the Python all() function with the help of examples. The all() function returns True if all elements in the given iterable are […]
In this tutorial, we will learn about the Python any() function with the help of examples. The any() function returns True if any element of an iterable is True. If […]
In this tutorial, we will learn about the Python abs() function with the help of examples. The abs() function returns the absolute value of the given number. […]
In this example, you will learn to convert bytes to a string. To understand this example, you should have the knowledge of the following Python programming topics: […]
In this example, you will learn to remove duplicate elements from a list. To understand this example, you should have the knowledge of the following Python […]
In this example, you will learn to count the number of occurrences of a character in a string. To understand this example, you should have […]
In this example, you will learn to create a countdown timer. To understand this example, you should have the knowledge of the following Python programming topics: Python […]