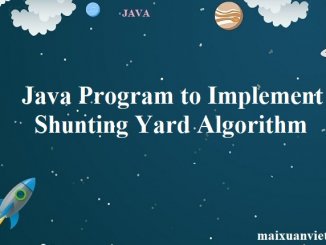
Java Program to Implement Shunting Yard Algorithm
This is a Java Program to Implement Shunting Yard Algorithm. Shunting Yard algorithm is used for converting an infix expression into a postfix expression. Here […]
This is a Java Program to Implement Shunting Yard Algorithm. Shunting Yard algorithm is used for converting an infix expression into a postfix expression. Here […]
This is a Java Program to Implement Cubic convergence 1/pi Algorithm. Cubic convergence is an algorithm used to calculate the value of 1/p. Here is […]
This is a Java Program to Implement Borwein Algorithm. Borwein’s algorithm is an algorithm devised by Jonathan and Peter Borwein to calculate the value of […]
This is a Java Program to Implement Nth Root Algorithm. This program is used to calculate n th root of a number x. Here is […]
This is a Java Program to Implement Horner Algorithm. Horner’s method is an efficient method for calculating polynomials.Here is the source code of the Java […]
This is the java implementation of performing Discrete Fourier Transform using Fast Fourier Transform algorithm. This class finds the DFT of N (power of 2) […]
This is the java implementation of performing Discrete Fourier Transform using Fast Fourier Transform algorithm. This class finds the DFT of N (power of 2) […]
This is the java implementation of Naive DFT approach over function. Formula for calculating the coefficient is X(k) = Sum(x(n)*cos(2*PI*k*n/N) – iSum(x(n)*sin(2*PI*k*n/N)) over 0 to […]
This is the java implementation of calculating coefficients of the given function performing the Discrete-Fourier Transform. Formula for calculating the coefficient is X(k) = Sum(x(n)*cos(2*PI*k*n/N) […]
This is java program to implement Knapsack problem using Dynamic programming.Given weights and values of n items, put these items in a knapsack of capacity […]
This is the java implementation of classic Bin-Packing algorithm. In the bin packing problem, objects of different volumes must be packed into a finite number […]
This is a java program to perform partition of an integer in all possible ways. Every partition when added should result in the given integer. […]
This is a java program to implement a standard fractional knapsack problem. It is an algorithmic problem in combinatorial optimization in which the goal is […]
This is java program to implement 0/1 Knapsack problem. The knapsack problem or rucksack problem is a problem in combinatorial optimization: Given a set of […]
This is a Java Program to Implement Knapsack Algorithm. This algorithm is used to solve knapsack problem : Given a set of items, each with […]
This is a java program to perform arithmetic operations on numbers which are greater than size of the integers. Here is the source code of […]
This is a sample program to multiply two given numbers using Schonhage-Strassen Algorithm. Suppose we are multiplying two numbers like 123 and 456 using long […]
This is a Java Program to Implement Karatsuba Multiplication Algorithm. The Karatsuba algorithm is a fast multiplication algorithm. It was discovered by Anatolii Alexeevitch Karatsuba […]
This is a Java Program to implement Booth Algorithm. This is a program to compute product of two numbers by using Booth’s Algorithm. This program […]
This is the java program to perform addition of two numbers without using any arithmetic operators. The summation of two numbers can be obtained using […]