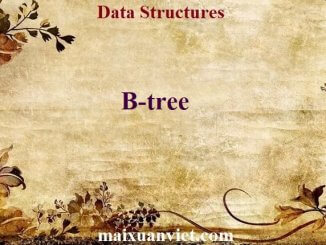
ALGORITHM
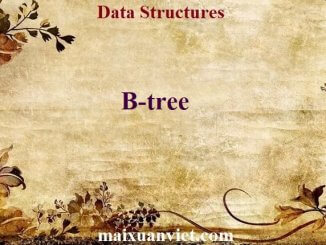
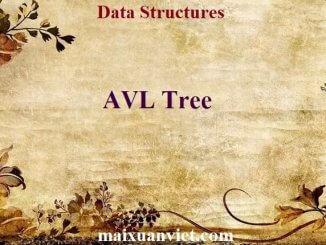
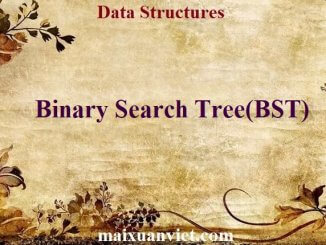
Binary Search Tree (BST)
1. Overview In this tutorial, you will learn how Binary Search Tree works. Also, you will find working examples of Binary Search Tree in C, […]
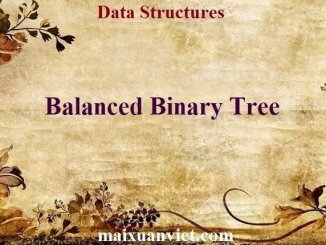
Balanced Binary Tree
1. Overview In this tutorial, you will learn about a balanced binary tree and its different types. Also, you will find working examples of a […]
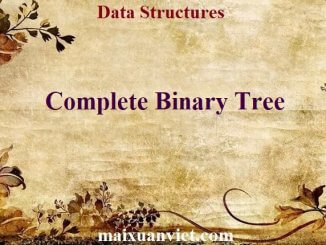
Complete Binary Tree
In this tutorial, you will learn about a complete binary tree and its different types. Also, you will find working examples of a complete binary […]
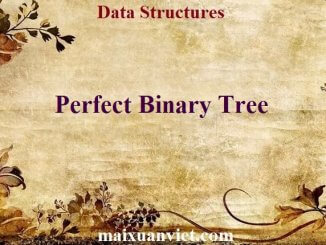
Perfect Binary Tree
1. Overview In this tutorial, you will learn about the perfect binary tree. Also, you will find working examples for checking a perfect binary tree […]
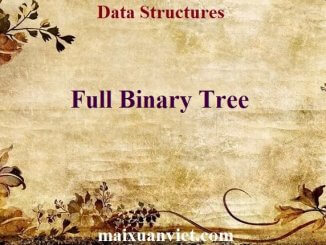
Full Binary Tree
In this tutorial, you will learn about full binary tree and its different theorems. Also, you will find working examples to check full binary tree […]
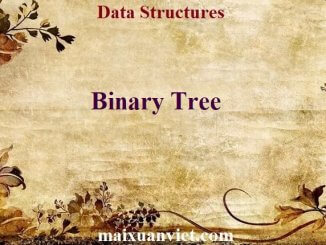
Binary Tree
In this tutorial, you will learn about binary tree and its different types. Also, you will find working examples of binary tree in C, C++, […]
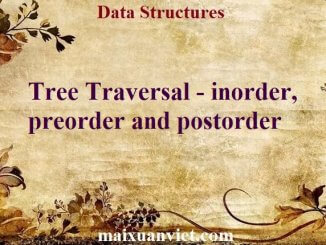
Tree Traversal – inorder, preorder and postorder
In this tutorial, you will learn about different tree traversal techniques. Also, you will find working examples of different tree traversal methods in C, C++, […]
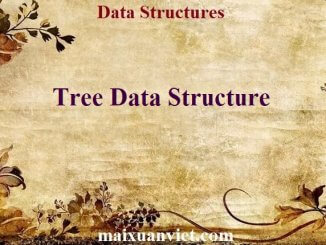
Tree Data Structure
In this tutorial, you will learn about tree data structure. Also, you will learn about different types of trees and the terminologies used in tree. […]
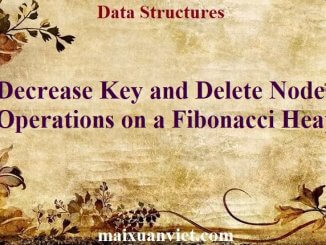
Decrease Key and Delete Node Operations on a Fibonacci Heap
In this tutorial, you will learn how decrease key and delete node operations work. Also, you will find working examples of these operations on a […]
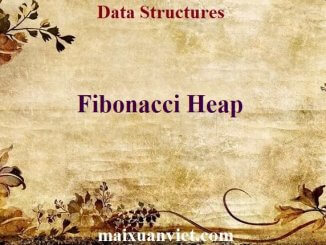
Fibonacci Heap
In this tutorial, you will learn what a Fibonacci Heap is. Also, you will find working examples of different operations on a fibonacci heap in […]
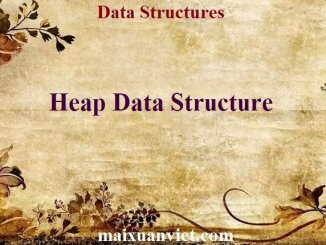
Heap Data Structure
In this tutorial, you will learn what heap data structure is. Also, you will find working examples of heap operations in C, C++, Java and […]
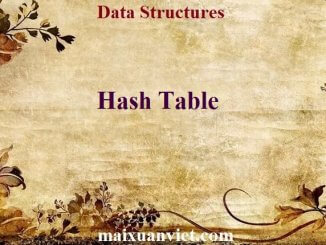
Hash Table
In this tutorial, you will learn what hash table is. Also, you will find working examples of hash table operations in C, C++, Java and […]
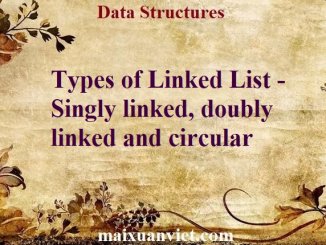
Types of Linked List – Singly linked, doubly linked and circular
In this tutorial, you will learn different types of linked list. Also, you will find implementation of linked list in C. Before you learn about […]
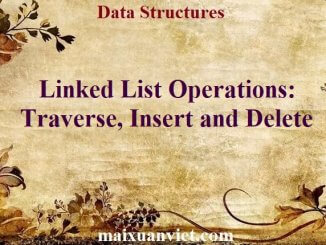
Linked List Operations: Traverse, Insert and Delete
1. Overview In this tutorial, you will learn different operations on a linked list. Also, you will find implementation of linked list operations in C/C++, […]
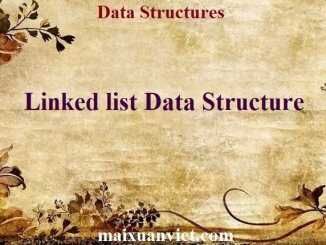
Linked list Data Structure
In this tutorial, you will learn about linked list data structure and it’s implementation in Python, Java, C, and C++. A linked list is a […]
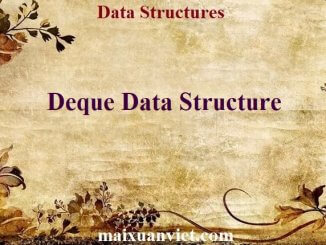
Deque Data Structure
In this tutorial, you will learn what a double ended queue (deque) is. Also, you will find working examples of different operations on a deque […]
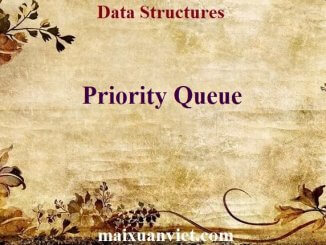
Priority Queue
In this tutorial, you will learn what priority queue is. Also, you will learn about it’s implementations in Python, Java, C, and C++. A priority […]
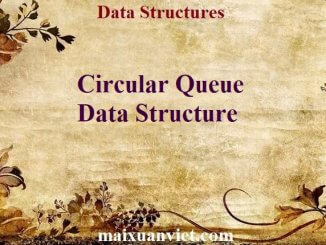
Circular Queue Data Structure
In this tutorial, you will learn what a circular queue is. Also, you will find implementation of circular queue in C, C++, Java and Python. […]