It’s the year 5555. You have a graph, and you want to find a long cycle and a huge independent set, just because you can. But for now, let’s just stick with finding either.
Given a connected graph with $n$ vertices, you can choose to either:
- find an independent set that has exactly $\lceil\sqrt{n}\rceil$ vertices.
- find a simple cycle of length at least $\lceil\sqrt{n}\rceil$.
An independent set is a set of vertices such that no two of them are connected by an edge. A simple cycle is a cycle that doesn’t contain any vertex twice. I have a proof you can always solve one of these problems, but it’s too long to fit this margin.
Input
The first line contains two integers $n$ and $m$ ($5 \le n \le 10^5$, $n-1 \le m \le 2 \cdot 10^5$) — the number of vertices and edges in the graph.
Each of the next $m$ lines contains two space-separated integers $u$ and $v$ ($1 \le u,v \le n$) that mean there’s an edge between vertices $u$ and $v$. It’s guaranteed that the graph is connected and doesn’t contain any self-loops or multiple edges.
Output
If you choose to solve the first problem, then on the first line print “1”, followed by a line containing $\lceil\sqrt{n}\rceil$ distinct integers not exceeding $n$, the vertices in the desired independent set.
If you, however, choose to solve the second problem, then on the first line print “2”, followed by a line containing one integer, $c$, representing the length of the found cycle, followed by a line containing $c$ distinct integers integers not exceeding $n$, the vertices in the desired cycle, in the order they appear in the cycle.
Examples
input
6 6 1 3 3 4 4 2 2 6 5 6 5 1
output
1 1 6 4
input
6 8 1 3 3 4 4 2 2 6 5 6 5 1 1 4 2 5
output
2 4 1 5 2 4
input
5 4 1 2 1 3 2 4 2 5
output
1 3 4 5
Note
In the first sample:
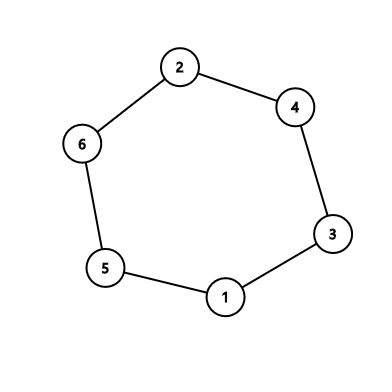
Notice that you can solve either problem, so printing the cycle $2-4-3-1-5-6$ is also acceptable.
In the second sample:
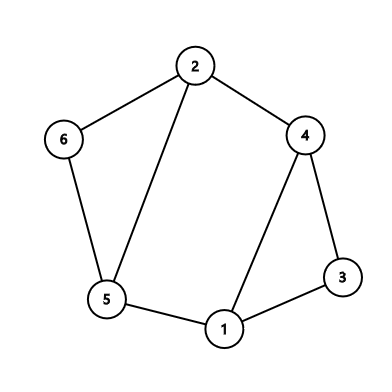
Notice that if there are multiple answers you can print any, so printing the cycle $2-5-6$, for example, is acceptable.
In the third sample:
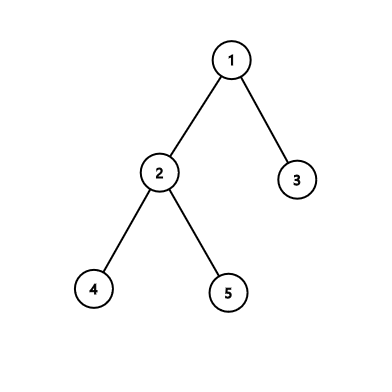
Solution:
#include <bits/stdc++.h> using namespace std; #define rep(i,a,n) for (int i=a;i<n;i++) #define per(i,a,n) for (int i=n-1;i>=a;i--) #define pb push_back #define mp make_pair #define all(x) (x).begin(),(x).end() #define fi first #define se second #define SZ(x) ((int)(x).size()) typedef vector<int> VI; typedef long long ll; typedef pair<int,int> PII; typedef double db; mt19937 mrand(random_device{}()); const ll mod=1000000007; int rnd(int x) { return mrand() % x;} ll powmod(ll a,ll b) {ll res=1;a%=mod; assert(b>=0); for(;b;b>>=1){if(b&1)res=res*a%mod;a=a*a%mod;}return res;} ll gcd(ll a,ll b) { return b?gcd(b,a%b):a;} // head const int N=101000; int dep[N],vis[N],par[N]; int n,m,u,v,d,cnt[N]; VI e[N]; void dfs(int u) { vis[u]=1; for (auto v:e[u]) { if (vis[v]&&dep[v]-dep[u]>=d-1) { VI cyc; int x=v; while (x!=u) { cyc.pb(x); x=par[x]; } cyc.pb(u); assert(SZ(cyc)>=d); puts("2"); printf("%d\n",SZ(cyc)); for (auto u:cyc) printf("%d ",u); puts(""); exit(0); } else if (!vis[v]) { dep[v]=dep[u]+1; par[v]=u; dfs(v); } } } int main() { scanf("%d%d",&n,&m); rep(i,0,m) { scanf("%d%d",&u,&v); e[u].pb(v); e[v].pb(u); } d=sqrt(n)-1; while (d*d<n) d+=1; dfs(1); rep(i,1,n+1) { cnt[dep[i]%(d-1)]++; } int p=max_element(cnt,cnt+d-1)-cnt; VI ind; rep(i,1,n+1) if (dep[i]%(d-1)==p) ind.pb(i); ind.resize(d); puts("1"); for (auto x:ind) printf("%d ",x); puts(""); }