Table of Contents
1. Giới thiệu
Java Print Service API (Dịch vụ in) cho phép thực hiện lệnh in trên tất cả các nền tảng Java. Java Print Service API bao gồm một bộ thuộc tính (attribute) in mở rộng dựa trên các thuộc tính chuẩn được chỉ định trong Giao thức Internet Printing Protocol (IPP). Với các thuộc tính này, ứng dụng client và server có thể khám phá và chọn các máy in có các tính năng được xác định bởi các thuộc tính. Ngoài ra StreamPrintService cho phép các ứng dụng có thể chuyển mã dữ liệu sang các định dạng khác nhau, các bên thứ ba có thể tự động cài đặt các dịch vụ in riêng của họ thông qua Service Provider Interface.
Java Print Service API bao gồm các package:
- javax.print: Cung cấp các lớp và interface chính cho Java Print Service API.
- javax.print.attribute: Cung cấp các lớp và interface mô tả các loại thuộc tính Java Print Service và các phương thức có thể được thu thập thành các bộ thuộc tính.
- javax.print.attribute.standard: Bao gồm các lớp xác định các thuộc tính in cụ thể.
- javax.print.event: Bao gồm các lớp sự kiện (event) và listener interfaces để theo dõi các dịch vụ in và tiến độ công việc in cụ thể.
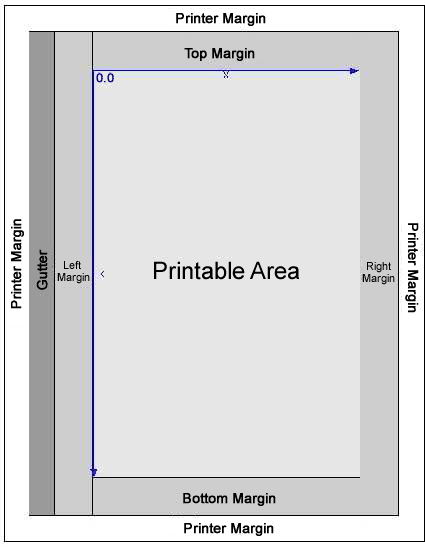
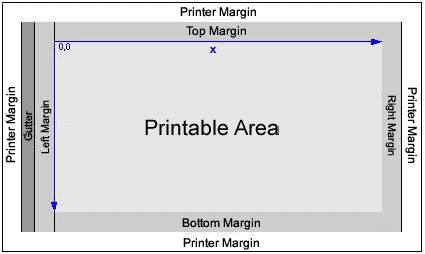
2. Ví dụ liệt kê danh sách dịch vụ in hiện có
import javax.print.PrintService; import java.awt.print.PrinterJob; public class PrintName { public static void main(String[] args) { // // Lookup for the available print services. // PrintService[] printServices = PrinterJob.lookupPrintServices(); // // Iterates the print services and print out its name. // for (PrintService printService : printServices) { String name = printService.getName(); System.out.println("Name = " + name); } } }
3. Ví dụ lấy dịch vụ in mặc định của hệ thống và hiển thị các thuộc tính của nó
import javax.print.PrintService; import javax.print.PrintServiceLookup; import javax.print.attribute.Attribute; import javax.print.attribute.AttributeSet; public class GetPrinter { public static void main(String[] args) { // // Gets the default print service for this environment. // Null is returned when no default print service found. // PrintService printer = PrintServiceLookup.lookupDefaultPrintService(); if (printer != null) { String printServiceName = printer.getName(); System.out.println("Print Service Name = " + printServiceName); // // Getting print service's attribute set. // AttributeSet attributes = printer.getAttributes(); for (Attribute a : attributes.toArray()) { String name = a.getName(); String value = attributes.get(a.getClass()).toString(); System.out.println(name + " : " + value); } } } }
Kết quả thực thi chương trình trên:
Print Service Name = Foxit Reader PDF Printer printer-name : Foxit Reader PDF Printer printer-is-accepting-jobs : accepting-jobs color-supported : supported queued-job-count : 0
4. Ví dụ tạo ứng dụng in file sử dụng Java Print Service
Một ứng dụng sử dụng Java Print Service API thực hiện các bước sau để xử lý một yêu cầu in:
- Tạo một lớp định nghĩa định dạng dữ liệu in (một DocFlavor phù hợp: pdf, text, …).
- Tạo và điền vào một AttributeSet, gói gọn một tập hợp các thuộc tính mô tả các khả năng dịch vụ in mong muốn, chẳng hạn như: khả năng in 5 bản sao, đóng dấu và hai mặt.
- Tìm một dịch vụ in có thể xử lý các yêu cầu in như được xác định bởi DocFlavor và tập hợp các thuộc tính.
- Tạo một lệnh in từ dịch vụ in (Java Print Service).
- Gọi phương thức in.
import java.io.BufferedInputStream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import javax.print.Doc; import javax.print.DocFlavor; import javax.print.DocPrintJob; import javax.print.PrintException; import javax.print.PrintService; import javax.print.PrintServiceLookup; import javax.print.SimpleDoc; import javax.print.attribute.HashPrintRequestAttributeSet; import javax.print.attribute.PrintRequestAttributeSet; import javax.print.attribute.standard.Copies; import javax.print.attribute.standard.MediaSize; import javax.print.attribute.standard.Sides; import javax.print.event.PrintJobAdapter; import javax.print.event.PrintJobEvent; public class PrintFileExample { private static boolean jobRunning = true; public static void main(String[] args) throws InterruptedException, IOException { // Input the file InputStream is = new BufferedInputStream(new FileInputStream("data/test.pdf")); // Set the document type: create a PDF doc flavor DocFlavor myFormat = DocFlavor.INPUT_STREAM.PDF; // Create a Doc Doc myDoc = new SimpleDoc(is, myFormat, null); // Build a set of attributes PrintRequestAttributeSet aset = new HashPrintRequestAttributeSet(); aset.add(new Copies(5)); // print five copies aset.add(MediaSize.ISO.A4); // print stapled aset.add(Sides.DUPLEX); // print double-sided // discover the printers that can print the format according to the // instructions in the attribute set PrintService[] services = PrintServiceLookup.lookupPrintServices(myFormat, aset); // Create a print job from one of the print services if (services.length > 0) { // Create and return a PrintJob capable of handling data from // any of the supported document flavors. DocPrintJob printJob = services[0].createPrintJob(); // register a listener to get notified when the job is complete printJob.addPrintJobListener(new JobCompleteMonitor()); try { // Print a document with the specified job attributes. printJob.print(myDoc, aset); while (jobRunning) { Thread.sleep(1000); } } catch (PrintException pe) { } } System.out.println("Exiting app"); is.close(); } private static class JobCompleteMonitor extends PrintJobAdapter { @Override public void printJobCompleted(PrintJobEvent jobEvent) { System.out.println("Job completed"); jobRunning = false; } } }