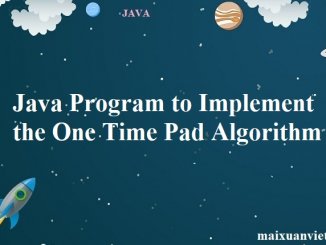
Java Program to Implement the One Time Pad Algorithm
This is a java program to implement one time pad algorithm. In cryptography, a one-time pad (OTP) is an encryption technique that cannot be cracked […]
This is a java program to implement one time pad algorithm. In cryptography, a one-time pad (OTP) is an encryption technique that cannot be cracked […]
This is a java program to implement Caesar Cipher Encryption algorithm. This is the simplest of all, where every character of the message is replaced […]
This is a java program to implement Wagner and Fisher Algorithm. In computer science, the Wagner–Fischer algorithm is a dynamic programming algorithm that computes the […]
This is a java program to implement Levenshtein Distance Computing Algorithm. In computer science, edit distance is a way of quantifying how dissimilar two strings […]
This is a java program to perform search using Vectors. Here is the source code of the Java Program to Implement String Matching Using Vectors. […]
This is a java program to implement grep linux command. Here is the source code of the Java Program to Implement the Program Used in […]
This is a java program to implement Aho-Corasick Algorithm. It is a kind of dictionary-matching algorithm that locates elements of a finite set of strings […]
This is a java program to search string using simple algorithm BoyerMoore. Here is the source code of the Java Program to Implement the String […]
This is a Java Program to Implement Bresenham Line Algorithm. The Bresenham line algorithm is an algorithm which determines which order to form a close […]
This is a Java Program to implement First fit Decreasing Bin Packing algorithm. The First Fit Decreasing (FFD) strategy, operates by first sorting the items […]
This is a Java Program to implement Lloyd’s Algorithm. The LBG-algorithm or Lloyd’s algorithm allows clustering of vectors of any dimension. This is helpful for […]
This is a Java Program to implement Quick Hull Algorithm to find convex hull. Here we’ll talk about the Quick Hull algorithm, which is one […]
This is a Java Program to Implement Jarvis Algorithm. Jarvis algorithm or the gift wrapping algorithm is an algorithm for computing the convex hull of […]
This is a Java Program to implement Gift Wrapping Algorithm. For the sake of simplicity, the description below assumes that the points are in general […]
This is a Java Program to implement Graham Scan Algorithm. Graham’s scan is a method of computing the convex hull of a finite set of […]
This is a Java Program to find the area of a polygon using slicker method. The algorithm assumes the usual mathematical convention that positive y […]
This Java Program is to Implement Knight’s Tour Problem.A knight’s tour is a sequence of moves of a knight on a chessboard such that the […]
This Java Program is to Implement Traveling Salesman Problem using Nearest neighbour Algorithm.The travelling salesman problem (TSP) or travelling salesperson problem asks the following question: […]
This is a java program to solve TSP using branch and bound algorithm. Branch and bound (BB or B&B) is an algorithm design paradigm for […]
This is a Java Program to Implement Hamiltonian Cycle Algorithm. Hamiltonian cycle is a path in a graph that visits each vertex exactly once and […]