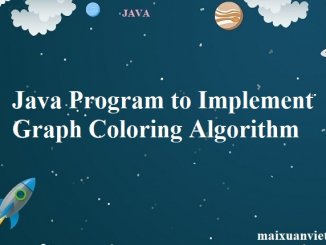
Java Program to Implement Graph Coloring Algorithm
This is a Java Program to Implement Graph Coloring Algorithm. Graph Coloring is a way of coloring the vertices of a undirected graph such that […]
This is a Java Program to Implement Graph Coloring Algorithm. Graph Coloring is a way of coloring the vertices of a undirected graph such that […]
This Java program Implements Maximum Length Chain Of Pairs.Given n pairs of numbers. In every pair, the first number is always smaller than the second […]
This Java program Implements Word Wrap Problem.A Given a sequence of words, and a limit on the number of characters that can be put in […]
This Java program is to Implement Network Flow problem. In graph theory, a flow network (also known as a transportation network) is a directed graph […]
This Java program is to Implement Max Flow Min Cut theorem. In optimization theory, the max-flow min-cut theorem states that in a flow network, the […]
This Java program is to Implement Ford-Fulkerson algorithm. The Ford–Fulkerson method (named for L. R. Ford, Jr. and D. R. Fulkerson) is an algorithm which […]
This Java program is to Implement Disjoint Sets. A disjoint-set data structure is a data structure that keeps track of a set of elements partitioned […]
This Java program,Implements Uniform Cost Search.In computer science, uniform-cost search (UCS) is a tree search algorithm used for traversing or searching a weighted tree, tree […]
This Java program,Implements Iterative Deepening.Iterative deepening depth-first search(IDDFS) is a state space search strategy in which a depth-limited search is run repeatedly, increasing the depth […]
This Java program,Implements Depth Limited Search.Like the normal depth-first search, depth-limited search is an uninformed search. It works exactly like depth-first search, but avoids its […]
This Java program,performs the DFS traversal on the given graph represented by a adjacency matrix to find all the back edges in a graph.the DFS […]
This Java program,performs the DFS traversal on the given graph represented by a adjacency matrix to find all the cross edges in a graph.the DFS […]
This Java program,performs the DFS traversal on the given graph represented by a adjacency matrix to find all the forward edges in a graph.Forward Edges […]
This Java program,implements Best-First Search.Best-first search is a search algorithm which explores a graph by expanding the most promising node chosen according to a specified […]
This is a Java Program to Implement Fenwick Tree. A Fenwick tree or binary indexed tree is a data structure providing efficient methods for calculation […]
This is a Java Program to implement Binary Search Tree using Linked Lists. A binary search tree (BST), sometimes also called an ordered or sorted […]
This is a Java Program to implement Expression Tree. Expression Tree is used in evaluating expressions. Here is the source code of the Java program […]
This is a Java Program to implement Self Balancing Binary Search Tree. A self-balancing (or height-balanced) binary search tree is any node-based binary search tree […]
This is a Java Program to Implement Ternary Search Tree. A ternary search tree is a type of prefix tree where nodes are arranged as […]
This is a Java Program to implement ScapeGoat Tree. A scapegoat tree is a self-balancing binary search tree which provides worst-case O(log n) lookup time, […]