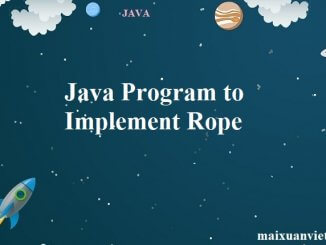
Java Program to Implement Rope
This is a Java Program to implement Rope. A rope is a data structure for storing and manipulating a very long string. Here is the […]
This is a Java Program to implement Rope. A rope is a data structure for storing and manipulating a very long string. Here is the […]
This is a Java Program to implement Red Black Tree. A red–black tree is a type of self-balancing binary search tree. The self-balancing is provided […]
This is a Java Program to implement Treap. Treap is a form of binary search tree data structure that maintain a dynamic set of ordered […]
This is a Java Program to implement Randomized Binary Search Tree. The randomized binary search tree stores the same nodes with the same random distribution […]
This is a Java Program to implement Suffix Tree. A suffix tree is a compressed trie containing all the suffixes of the given text as […]
This is a Java Program to implement Trie. A trie is an ordered tree data structure that is used to store a dynamic set or […]
This is a Java Program to implement Weight Balanced Tree. A weight-balanced binary tree is a binary tree which is balanced based on knowledge of […]
This is a Java Program to implement Threaded Binary Tree. A threaded binary tree makes it possible to traverse the values in the binary tree […]
This is a Java Program to implement Splay Tree. A splay tree is a self-adjusting binary search tree with the additional property that recently accessed […]
This is a Java Program to implement AVL Tree. An AVL tree is a self-balancing binary search tree, and it was the first such data […]
This is a Java Program to implement AA Tree. An AA tree is a form of balanced tree used for storing and retrieving ordered data […]
This is a Java Program to implement Cartesian Tree. A Cartesian tree is a binary tree derived from a sequence of numbers. It can be […]
This is a Java Program to implement Binary Search Tree. A binary search tree (BST), sometimes also called an ordered or sorted binary tree, is […]
This is a Java Program to implement Binary Tree. A binary tree is a tree data structure in which each node has at most two […]
This Java program is to Implement ternary tree. In computer science, a ternary tree is a tree data structure in which each node has at […]
This is a java program to implement Range Tree. A range tree on a set of 1-dimensional points is a balanced binary search tree on […]
This is a java program to implement Interval Tree. In computer science, an interval tree is an ordered tree data structure to hold intervals. Specifically, […]
This Java program is to Implement Segment tree. In computer science, a segment tree is a tree data structure for storing intervals, or segments. It […]
This is a Java Program to perform Double Order traversal over binary tree.Recurse through:1. Visit root of (sub)tree.2. Visit left sub-tree.3. Revisit root of (sub)tree.4. […]
This is a java program to find global min cut of the graph. In computer science and graph theory, Karger’s algorithm is a randomized algorithm […]