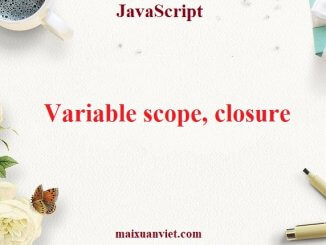
JavaScript Variable scope, closure
JavaScript is a very function-oriented language. It gives us a lot of freedom. A function can be created at any moment, passed as an argument […]
JavaScript is a very function-oriented language. It gives us a lot of freedom. A function can be created at any moment, passed as an argument […]
Many JavaScript built-in functions support an arbitrary number of arguments. For instance: Math.max(arg1, arg2, …, argN) – returns the greatest of the arguments. Object.assign(dest, src1, …, […]
Let’s return to functions and study them more in-depth. Our first topic will be recursion. If you are not new to programming, then it is probably […]
Let’s say we have a complex object, and we’d like to convert it into a string, to send it over a network, or just to […]
Let’s meet a new built-in object: Date. It stores the date, time and provides methods for date/time management. For instance, we can use it to store […]
Let’s step away from the individual data structures and talk about the iterations over them. In the previous chapter we saw methods map.keys(), map.values(), map.entries(). These methods are […]
1. Overview As we know from the chapter Garbage collection, JavaScript engine keeps a value in memory while it is “reachable” and can potentially be used. […]
Till now, we’ve learned about the following complex data structures: Objects are used for storing keyed collections. Arrays are used for storing ordered collections. But […]
Iterable objects are a generalization of arrays. That’s a concept that allows us to make any object useable in a for..of loop. Of course, Arrays are iterable. But […]
Arrays provide a lot of methods. To make things easier, in this chapter they are split into groups. 1. Add/remove items We already know methods […]
Objects allow you to store keyed collections of values. That’s fine. But quite often we find that we need an ordered collection, where we have a […]
In JavaScript, the textual data is stored as strings. There is no separate type for a single character. The internal format for strings is always UTF-16, […]
In modern JavaScript, there are two types of numbers: Regular numbers in JavaScript are stored in 64-bit format IEEE-754, also known as “double precision floating point […]
1. Overview JavaScript allows us to work with primitives (strings, numbers, etc.) as if they were objects. They also provide methods to call as such. […]
A recent additionThis is a recent addition to the language. Old browsers may need polyfills. The optional chaining ?. is a safe way to access nested object […]
The regular {…} syntax allows to create one object. But often we need to create many similar objects, like multiple users or menu items and so on. […]
Objects are usually created to represent entities of the real world, like users, orders and so on: And, in the real world, a user can act: […]
Memory management in JavaScript is performed automatically and invisibly to us. We create primitives, objects, functions… All that takes memory. What happens when something is […]
1. Overview One of the fundamental differences of objects versus primitives is that objects are stored and copied “by reference”, whereas primitive values: strings, numbers, […]
As we know from the chapter Data types, there are eight data types in JavaScript. Seven of them are called “primitive”, because their values contain only […]