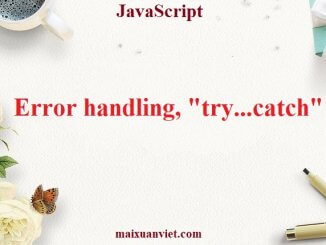
JavaScript Error handling, “try…catch”
No matter how great we are at programming, sometimes our scripts have errors. They may occur because of our mistakes, an unexpected user input, an […]
No matter how great we are at programming, sometimes our scripts have errors. They may occur because of our mistakes, an unexpected user input, an […]
In JavaScript we can only inherit from a single object. There can be only one [[Prototype]] for an object. And a class may extend only one other […]
The instanceof operator allows to check whether an object belongs to a certain class. It also takes inheritance into account. Such a check may be necessary in […]
1. Overview Built-in classes like Array, Map and others are extendable also. For instance, here PowerArray inherits from the native Array: Please note a very interesting thing. Built-in […]
One of the most important principles of object oriented programming – delimiting internal interface from the external one. That is “a must” practice in developing […]
Class inheritance is a way for one class to extend another class. So we can create new functionality on top of the existing. 1. The […]
In object-oriented programming, a class is an extensible program-code-template for creating objects, providing initial values for state (member variables) and implementations of behavior (member functions or methods). […]
The “prototype” property is widely used by the core of JavaScript itself. All built-in constructor functions use it. First we’ll see at the details, and then how […]
1. Overview Remember, new objects can be created with a constructor function, like new F(). If F.prototype is an object, then the new operator uses it to set [[Prototype]] for the new […]
In programming, we often want to take something and extend it. For instance, we have a user object with its properties and methods, and want to make admin and guest as […]
There are two kinds of object properties. The first kind is data properties. We already know how to work with them. All properties that we’ve been […]
As we know, objects can store properties. Until now, a property was a simple “key-value” pair to us. But an object property is actually a […]
Let’s revisit arrow functions. Arrow functions are not just a “shorthand” for writing small stuff. They have some very specific and useful features. JavaScript is […]
When passing object methods as callbacks, for instance to setTimeout, there’s a known problem: “losing this“. In this chapter we’ll see the ways to fix it. 1. […]
JavaScript gives exceptional flexibility when dealing with functions. They can be passed around, used as objects, and now we’ll see how to forward calls between them and decorate them. […]
We may decide to execute a function not right now, but at a certain time later. That’s called “scheduling a call”. There are two methods […]
There’s one more way to create a function. It’s rarely used, but sometimes there’s no alternative. 1. Syntax The syntax for creating a function: The […]
As we already know, a function in JavaScript is a value. Every value in JavaScript has a type. What type is a function? In JavaScript, […]
1. Overview The global object provides variables and functions that are available anywhere. By default, those that are built into the language or the environment. […]
This article is for understanding old scripts The information in this article is useful for understanding old scripts. That’s not how we write a new […]