Table of Contents
1. Overview
The difference() method returns the set difference of two sets.
If A and B are two sets. The set difference of A and B is a set of elements that exists only in set A but not in B. For example:
If A = {1, 2, 3, 4} B = {2, 3, 9} Then, A - B = {1, 4} B - A = {9}
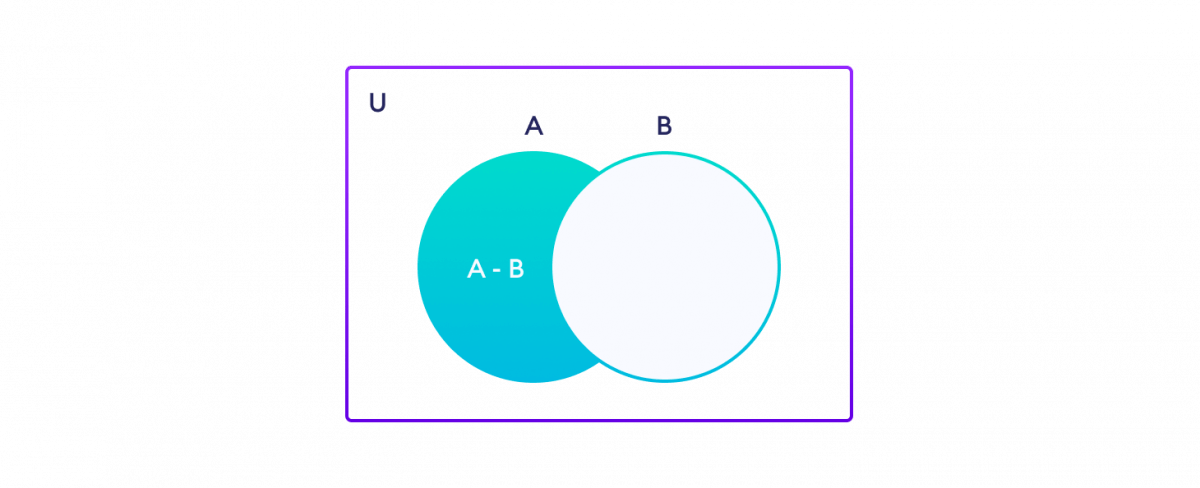
The syntax of the set difference()
method in Python is:
A.difference(B)
Here, A and B are two sets. The following syntax is equivalent to A-B
.
2. Return Value from difference()
difference()
returns the difference between two sets which is also a set. It doesn’t modify the original sets.
3. Example 1: How difference() works in Python?
A = {'a', 'b', 'c', 'd'} B = {'c', 'f', 'g'} # Equivalent to A-B print(A.difference(B)) # Equivalent to B-A print(B.difference(A))
Output
{'b', 'a', 'd'} {'g', 'f'}
You can also find the set difference using -
operator in Python.
4. Example 2: Set Difference Using – Operator.
A = {'a', 'b', 'c', 'd'} B = {'c', 'f', 'g'} print(A-B) print(B-A)
Output
{'b', 'd', 'a'} {'f', 'g'}
Related posts:
Node.js vs Python for Backend Development
Python map()
Python Program to Find the Factorial of a Number
Python Machine Learning Cookbook - Practical solutions from preprocessing to Deep Learning - Chris A...
Python Program to Check If Two Strings are Anagram
Python Global, Local and Nonlocal variables
Python List clear()
Applied Text Analysis with Python - Benjamin Benfort & Rebecca Bibro & Tony Ojeda
Python object()
Python Program to Display Fibonacci Sequence Using Recursion
Python String join()
Python if...else Statement
Python Strings
Converting Between an Array and a Set in Java
Python Program to Convert Celsius To Fahrenheit
Python Set remove()
Python String startswith()
Python String isalpha()
Python Program to Append to a File
Python memoryview()
Deep Learning in Python - LazyProgrammer
Python Directory and Files Management
Python frozenset()
Python Program to Extract Extension From the File Name
Python Function Arguments
Python String isalnum()
Python exec()
Python Program to Convert Two Lists Into a Dictionary
Python compile()
Python String index()
Python Inheritance
Python Set add()