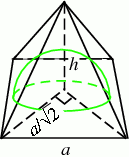
Input
The input contains a single floating-point number x with exactly 6 decimal places (0 < x < 5).
Output
Output two integers separated by a single space. Each integer should be between 1 and 10, inclusive. If several solutions exist, output any of them. Solution will exist for all tests.
Examples
input
1.200000
output
3 2
input
2.572479
output
10 3
input
4.024922
output
9 9
Solution:
#include <iostream> #include <iomanip> #include <cstdio> #include <set> #include <vector> #include <map> #include <cmath> #include <algorithm> #include <memory.h> #include <string> #include <cstring> #include <sstream> #include <cstdlib> #include <ctime> #include <cassert> using namespace std; int main() { double x; scanf("%lf", &x); double diff = 12345; int da = -1, db = -1; for (int a = 1; a <= 10; a++) for (int b = 1; b <= 10; b++) { double u = (a * b) / sqrt(4 * a * a + b * b); u = fabs(u - x); if (u < diff) { diff = u; da = b; db = a; } } printf("%d %d\n", da, db); return 0; }
Related posts:
Suffix Tree. Ukkonen's Algorithm
Pudding Monsters
Tape
Wise Men (Easy Version)
Increase Sequence
Network Configuration
Disjoint Set Union
Substitutes in Number
Little Artem and Random Variable
Bookshelves
Valid BFS?
Wilbur and Array
Giáo trình Thuật toán - Ngọc Anh Thư
Voting for Photos
Kuroni and the Score Distribution
Adding Powers
Bribes
K-th order statistic in O(N)
Golden System
Stairs and Elevators
A Serial Killer
To Hack or not to Hack
Kuroni and the Gifts
International Olympiad
Graph Coloring
Half-plane intersection - S&I Algorithm in O(Nlog N)
University Classes
Montgomery Multiplication
Little Artem
Auto completion
Red Blue Tree
Search for connected components in a graph