Table of Contents
Environment Variables are variables that are set by the Operating System. They are decoupled from application logic. They can be accessed from applications and programs through various APIs.
There is a Node.js library called dotenv
that helps you manage and load environment variables. Let’s take a look at the purpose of environment variables, how to use them, and their role in a development environment.
1. Purpose of Environment Variables
Environment Variables are important to a software developer for multiple reasons.
- Separation of Concerns
- Protecting Config Keys
1.1. Separation of Concerns
Separation of Concerns refers to a software design principle that states that computer programs should be divided into distinct sections, such that each section addresses a separate concern. This means that a developer working on one section needs to have minimal knowledge about other sections. The details of other sections are placed on the sideline.
Application Configuration is a section of the code that should be decoupled from the application. Good software practices state that app config requires strict separation of config from code. Such config files can be stored as environment variables.
1.2. Protecting Config Keys
With the increasing popularity of cloud computing, more applications are using cloud services and other external APIs. Most of these come with config keys for control and access management. If the API keys are added to the application, and the code is pushed to a public repository on GitHub, this could lead to an unmonitored access problem. Unknown parties might end up using your API keys, leading to an unintended bill for your cloud services, and other potential security issues.
To solve this problem, the config keys can be added as environment variables and invoked from a closed environment from where the application is deployed.
2. Environment Variables in Node.js
In Node.js, process.env
is a global variable that is injected during runtime. It is a view of the state of the system environment variables. When we set an environment variable, it is loaded into process.env
during runtime and can later be accessed.
dotenv
is a module available on npm to load environment variables into process.env
. dotenv
can be added to your Node.js project by installing it from npm or yarn:
# with npm npm install dotenv # or with Yarn yarn add dotenv
In a production environment, the IP address and the port on which it runs might change every single time, depending on the server. Since we cannot hardcode the server port, we can solve it by using dotenv
.
Create a file called index.js
and add the following code to it.
const express = require("express"); require("dotenv").config(); const app = express(); const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on the port ${port}.`); });
Suppose we want to use sensitive credentials like username and password in an open-source project, we can use dotenv
for that as well.
require("dotenv").config(); const mysql = require("mysql"); let con = mysql.createConnection({ host: process.env.DB_HOST, user: process.env.DB_USER, password: process.env.DB_PASS, });
Now, to set the env variables, create a .env
file at the root of the project directory.
DB_HOST=localhost DB_USER=admin DB_PASS=password
Enter the values of the following variables. This will load these variables into process.env
. We can add this .env
file to .gitignore
so that our credentials are protected.
3. Environment Variables in Deployment
When deploying to services like MaiXuanViet.com, Vercel, Netlify or Heroku, environment variables can be set so that our deployed apps can access them.
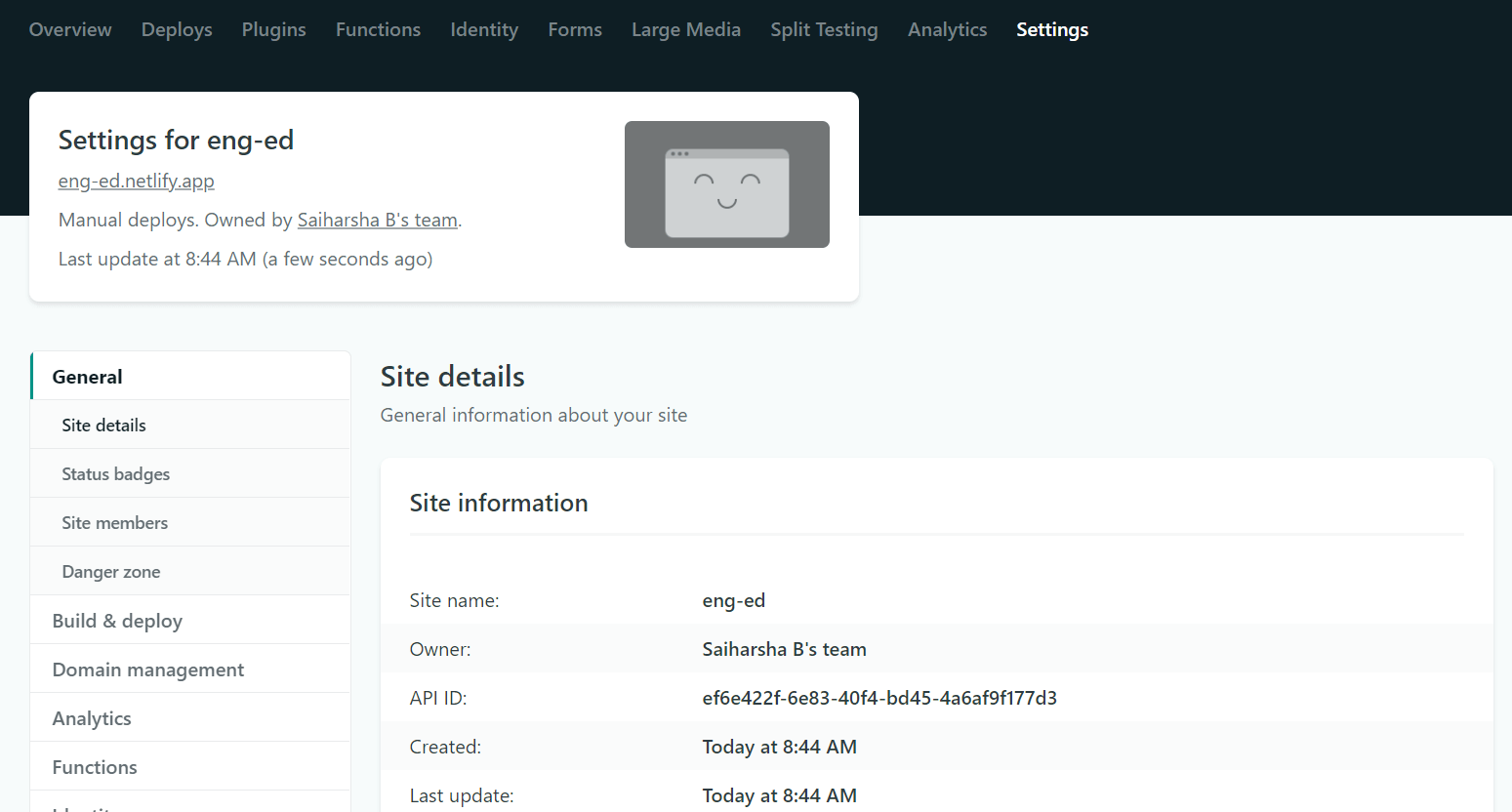
Using Netlify as an example, open the Netlify Dashboard of the app you’re about to deploy.
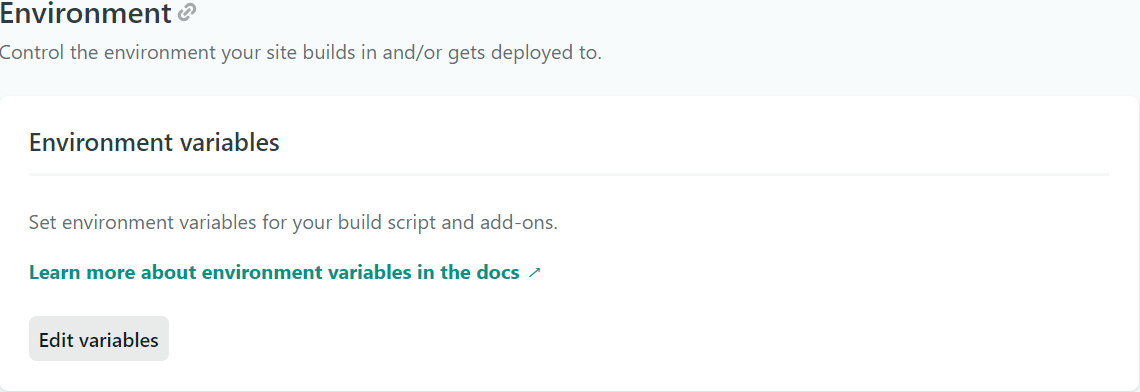
Go to Build and Deploy -> Environment Variables
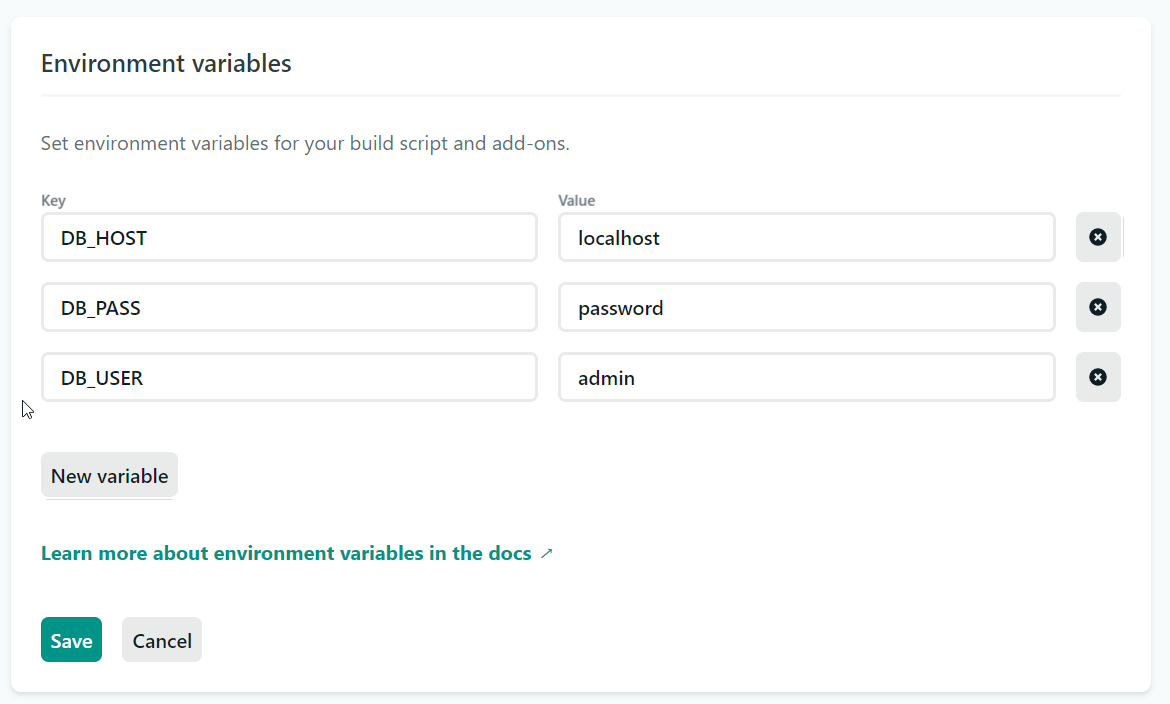
Now, set the variables and save.
Your environment variables are successfully set for deployment on Netlify.
4. Further Reading
- For detailed documentation and advanced configuration options for the
dotenv
module, visit the GitHub Page. - Learn how to use environment variables with the Nuxt Framework.
- Read about good software development practices at The Twelve Factor App.